Concepts of true, false, truthy and falsy in JavaScript
true and false are boolean data types in JavaScript, used to perform various logical operations and condition checks. Below is a detailed discussion of the usage of true and false, and the truthy and falsy values in JavaScript.
1. True and False are used when:
True:
The value true indicates that the result of a condition or logical test is "true".
False:
The value false indicates that any condition or logical test results in "false".
Example:
let isAdult = true; // একজন প্রাপ্তবয়স্ক হলে 'true' হবে let isStudent = false; // শিক্ষার্থী না হলে 'false' হবে if (isAdult) { console.log("You are an adult."); } else { console.log("You are not an adult."); }
2. Truthy and Falsy value keys:
Some values in JavaScript behave as true and false, even though they are not directly true or false. It has two categories:
Falsy values:
Falsy values are values that evaluate to "false" in a logical test. The following values are considered falsy in JavaScript:
- false
- 0 (zero)
- -0 (negative zero)
- "" (empty string)
- null
- undefined
- NaN (Not a Number)
Example:
if (0) { console.log("This will not run because 0 is falsy."); } else { console.log("Falsy value."); // এই লাইনটি রান করবে }
Truthy values:
Truthy values are values that evaluate to "true" in a logical test. Below are examples of some common truthy values:
- true
- Any non-zero number (eg: 1, -1, 100)
- Any non-empty string (eg: "hello", "false", "0")
- object (eg: {}, [])
- function
Example:
let isAdult = true; // একজন প্রাপ্তবয়স্ক হলে 'true' হবে let isStudent = false; // শিক্ষার্থী না হলে 'false' হবে if (isAdult) { console.log("You are an adult."); } else { console.log("You are not an adult."); }
3. Truthy and Falsy checking rule:
When you use a variable in an if or logical operation, JavaScript automatically evaluates it as truthy or falsy.
Truthy example:
if (0) { console.log("This will not run because 0 is falsy."); } else { console.log("Falsy value."); // এই লাইনটি রান করবে }
Falsy example:
if ("hello") { console.log("This will run because 'hello' is truthy."); // এই লাইনটি রান করবে } else { console.log("Falsy value."); }
4. for short:
- Truthy: Any value that evaluates to true when the condition is checked (eg: non-zero number, non-empty string, object).
- Falsy: Any value that is considered false in the condition check (eg: false, 0, "", null, undefined, NaN).
True and false conditions in JavaScript are important for making logical decisions. Understanding Truthy and Falsy will give you more skill in creating terms.
The above is the detailed content of Concepts of true, false, truthy and falsy in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










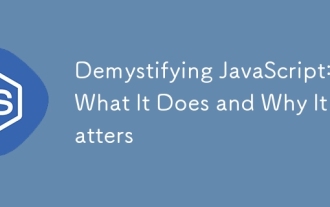
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
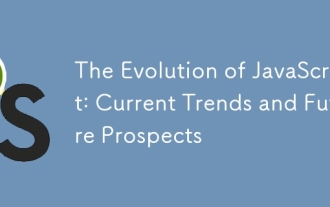
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
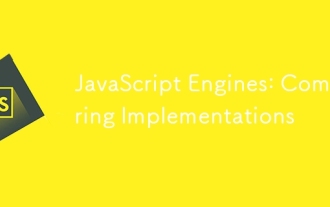
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
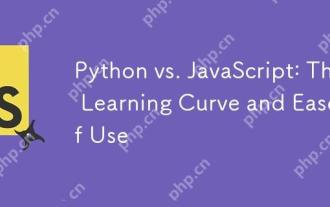
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
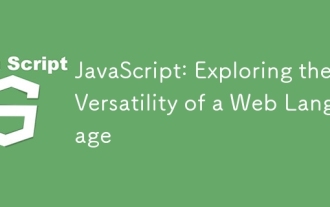
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
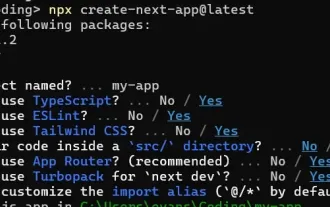
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
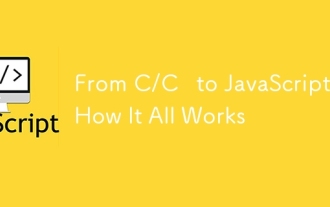
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
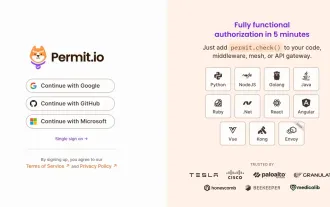
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
