


How can I pretty-print JSON in JavaScript for better readability and visual appeal?
Pretty-printing JSON in JavaScript for Improved Readability
When working with JSON data, it's often desirable to display it in a visually appealing manner for human readability. JavaScript provides a solution to this need with the JSON.stringify() method.
Using JSON.stringify() for Pretty-printing
By default, JSON.stringify() generates a compact string representation of the JSON object. However, you can enable pretty-printing by providing a third argument that specifies the spacing level.
var str = JSON.stringify(obj, null, 2); // spacing level = 2
This generates a JSON string with indentation and whitespace, making it easier to read and understand.
Syntax Highlighting for Enhanced Visual Appeal
If you desire additional visual enhancements, such as syntax highlighting, you can employ regex magic to achieve this:
function syntaxHighlight(json) { // Replace special characters for HTML safety json = json.replace(/&/g, '&amp;').replace(/</g, '&lt;').replace(/>/g, '&gt;'); // Add HTML spans with appropriate classes for styling return json.replace(/("(\u[a-zA-Z0-9]{4}|\[^u]|[^\"])*"(\s*:)?|\b(true|false|null)\b|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?)/g, function (match) { var cls = 'number'; // Default class for numbers if (/^"/.test(match)) { // String if (/:$/.test(match)) { // Key cls = 'key'; } else { // String value cls = 'string'; } } else if (/true|false/.test(match)) { // Boolean cls = 'boolean'; } else if (/null/.test(match)) { // Null cls = 'null'; } return '<span class="' + cls + '">' + match + '</span>'; }); }
Sample Code
Here's a full example that demonstrates both pretty-printing and syntax highlighting:
// Sample JSON object var obj = { a: 1, 'b': 'foo', c: [false, 'false', null, 'null', { d: { e: 1.3e5, f: '1.3e5' } }] }; // Pretty-print the JSON using `JSON.stringify()` var str = JSON.stringify(obj, undefined, 4); output(str); // Syntax highlight the pretty-printed JSON output(syntaxHighlight(str)); // Function to append output to the page function output(inp) { document.body.appendChild(document.createElement('pre')).innerHTML = inp; }
This code generates a visually appealing representation of the JSON object, making it easier for humans to read and comprehend.
The above is the detailed content of How can I pretty-print JSON in JavaScript for better readability and visual appeal?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
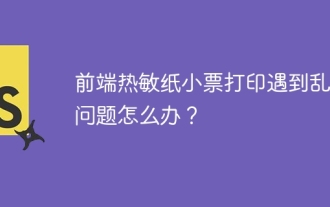
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
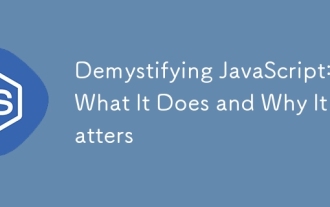
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
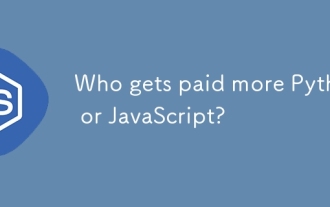
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
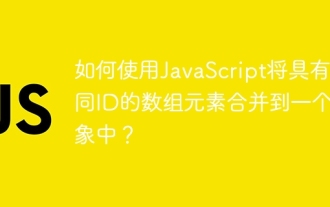
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
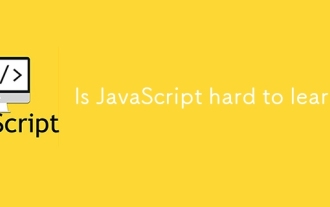
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
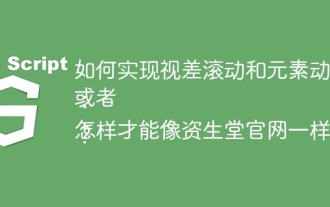
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
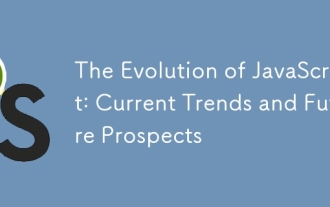
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
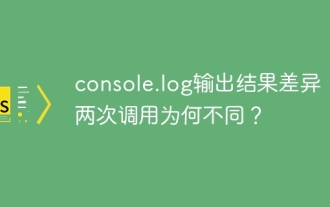
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
