


Why is my Go `reader.ReadString` function not correctly processing user input?
Troubleshooting "reader.ReadString" in Go
In Go, bufio.Reader provides the ReadString method to read a line of input from a stream, stopping at a specified delimiter character. However, when using this method to retrieve user input, it can sometimes be observed that the first occurrence of the delimiter is not stripped from the returned string.
Consider the following code:
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Who are you? \nEnter your name: ") text, _ := reader.ReadString('\n') if aliceOrBob(text) { fmt.Printf("Hello, ", text) } else { fmt.Printf("You're not allowed in here! Get OUT!!") } } func aliceOrBob(text string) bool { if text == "Alice" { return true } else if text == "Bob" { return true } else { return false } }
When this program runs, it prompts the user to enter their name, but even if the user enters "Alice" or "Bob", the program incorrectly prints "You're not allowed in here! Get OUT!!".
The problem lies in the fact that the ReadString method reads the line of input until the specified delimiter is encountered. However, in its default behavior, it does not remove the delimiter from the returned string. This means that when the user enters "Alice", the value of text will actually be "Alicen", which does not match any of the names in the aliceOrBob function.
To fix this, one can either use strings.TrimSpace to remove the newline character from text before checking it in the aliceOrBob function:
import ( .... "strings" .... ) ... if aliceOrBob(strings.TrimSpace(text)) { ...
Alternatively, one can use the ReadLine method instead of ReadString to read a line of input from os.Stdin. ReadLine returns a byte slice representing the line, without the newline character. However, since aliceOrBob expects a string, one will need to convert the byte slice to a string using the string function:
... text, _, _ := reader.ReadLine() if aliceOrBob(string(text)) { ...
The above is the detailed content of Why is my Go `reader.ReadString` function not correctly processing user input?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










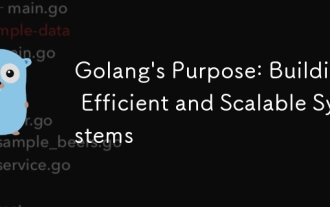
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
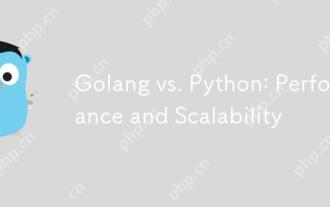
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
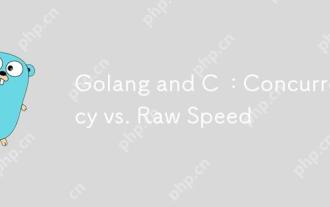
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
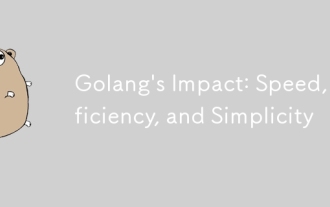
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
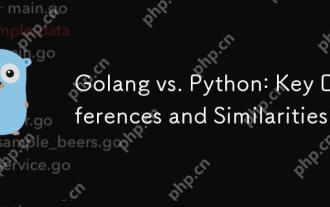
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
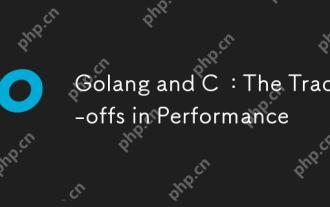
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
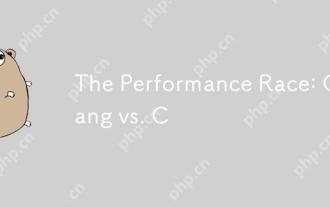
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
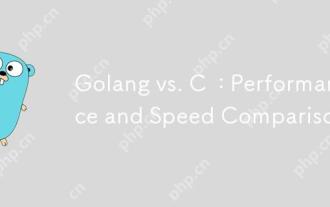
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
