


How Can We Implement an Either Type in Go, Given the Limitations of Generics in Interface Methods?
Implementing an Either Type in Go: Navigating Generics' Boundaries
The Problem and the Goal
With generics now a part of Go 1.18, many developers have set their sights on creating types that accurately represent complex concepts. A classic case is the 'Either[A, B]' type, which expresses the possibility of a value being one of two distinct types, A or B.
Interface Definition Hurdles
In defining an interface for an 'Either' type, a hurdle emerges due to the language's limitation of not allowing type parameters in interface methods. This restriction prevents us from expressing the 'Switch' method, which returns different types based on the value's variant.
A Creative Implementation
To overcome this issue, one could draw inspiration from functional programming languages and implement an 'Optional' type that encapsulates the ability to represent either a specific value or an absence. Building upon this, we can define the 'Either' type:
type Either[A, B any] interface { is_left() bool is_right() bool find_left() Optional[A] find_right() Optional[B] }
Instead of relying on a 'Switch' method, this approach leverages the 'is_left' and 'is_right' methods to determine the type of the contained value. The 'find_left' and 'find_right' methods then provide an 'Optional' value for the corresponding type.
Implementation Details and Usage
Within the 'Left' and 'Right' concrete types implementing 'Either,' we manage the actual payload and provide the necessary boolean flags. The 'left' and 'right' functions act as constructors, simplifying instantiation.
A sample usage demonstrates how to work with the 'Either' type:
func main() { var e1 Either[int, string] = left[int, string](4143) var e2 Either[int, string] = right[int, string]("G4143") if e1.is_left() { if l, err := e1.find_left().get(); err == nil { fmt.Printf("The int is: %d\n", l) } else { fmt.Fprintln(os.Stderr, err) } } }
Conclusion
While Go's generic limitations pose challenges, it's possible to work around them and create powerful abstractions like the 'Either' type. By leveraging 'Optional' and implementing a custom interface with helper methods, developers can gain the flexibility and expressiveness they seek in their Go code.
The above is the detailed content of How Can We Implement an Either Type in Go, Given the Limitations of Generics in Interface Methods?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


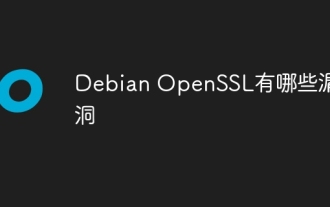
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
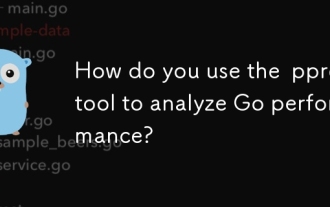
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
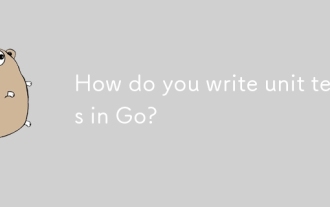
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
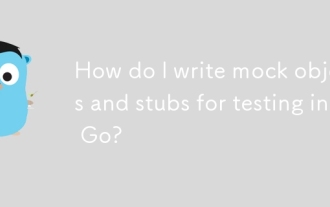
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
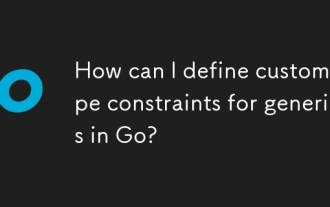
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
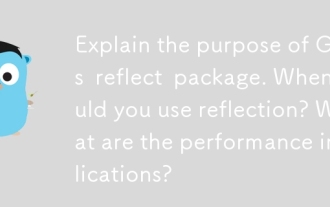
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
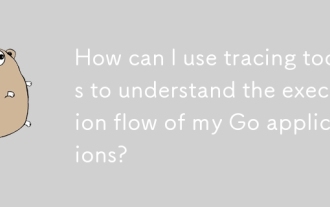
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
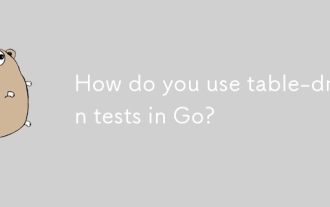
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
