


How Can I Efficiently Monitor File Changes in Python Without Polling?
Monitoring File Changes Without Polling Using Python
Problem:
You have a log file written by another process that you want to monitor for changes. Whenever a change occurs, you need to read the new data for processing.
Background:
You were considering using the PyWin32 library's win32file.FindNextChangeNotification function, but you are unsure how to configure it to watch a specific file.
Solution: Introducing Watchdog
Instead of polling, a more efficient option is to use the Watchdog library. Here's how:
import watchdog.observers import watchdog.events class MyEventHandler(watchdog.events.FileSystemEventHandler): def on_modified(self, path, metadata): # Read the modified log file and perform necessary processing # Create a Watchdog observer observer = watchdog.observers.Observer() # Add the log file path to be watched observer.schedule(MyEventHandler(), "path/to/log_file") # Start observing observer.start() # Wait indefinitely for changes observer.join()
Note:
If you are monitoring a file over a mapped network drive, Windows may not "hear" updates to the file as it does on a local disk. Consider using a different approach in such scenarios.
The above is the detailed content of How Can I Efficiently Monitor File Changes in Python Without Polling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


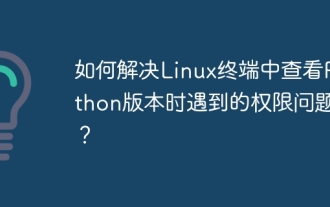
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
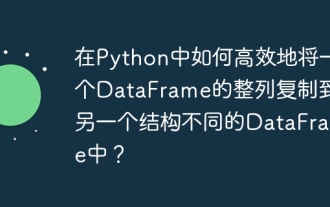
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
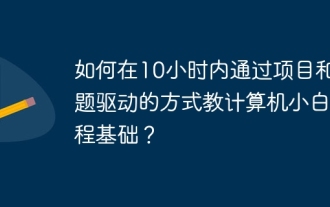
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
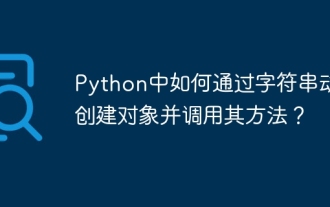
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
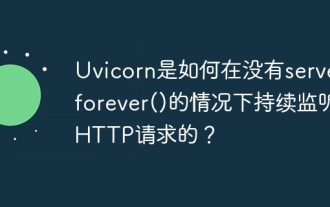
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
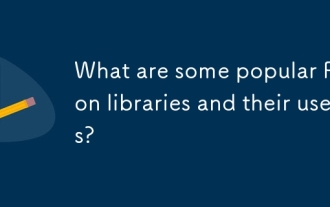
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
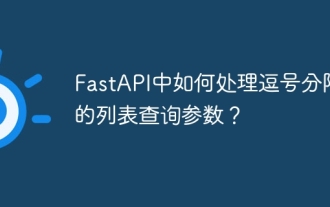
Fastapi ...
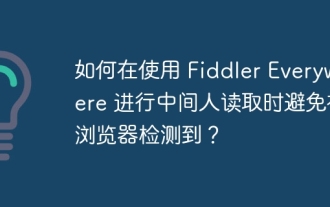
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
