How to Achieve 4 FLOPs Per Cycle on Modern x86-64 Intel CPUs?
How to achieve the theoretical maximum of 4 FLOPs per cycle?
It is theoretically possible to achieve a peak performance of 4 floating-point operations (double precision) per cycle on modern x86-64 Intel CPUs, by utilizing the following techniques:
Optimizing Code for SSE instructions
- Use SSE (Streaming SIMD Extensions) instructions, which enable parallel processing of multiple data elements.
- Ensure that the code is properly aligned for optimal SSE performance.
Loop unrolling and interleaving
- Unroll inner loops to improve instruction-level parallelism.
- Interleave multiplies and adds to take advantage of the CPU's pipelining capabilities.
Grouping operations in threes
- Arrange operations in groups of three to match the execution units on some Intel CPUs. This allows for alternating between add and mul instructions, maximizing throughput.
Avoiding unnecessary stalls and dependencies
- Minimize data dependencies between instructions to avoid stalls.
- Use compiler optimizations (-O3 or higher) to help identify and eliminate unnecessary dependencies.
Example code
The following code snippet demonstrates how to achieve close to peak performance on Intel Core i5 and Core i7 CPUs:
#include <emmintrin.h> #include <omp.h> #include <iostream> using namespace std; typedef unsigned long long uint64; double test_dp_mac_SSE(double x, double y, uint64 iterations) { register __m128d r0, r1, r2, r3, r4, r5, r6, r7, r8, r9, rA, rB, rC, rD, rE, rF; // Generate starting data. r0 = _mm_set1_pd(x); r1 = _mm_set1_pd(y); r8 = _mm_set1_pd(-0.0); r2 = _mm_xor_pd(r0, r8); r3 = _mm_or_pd(r0, r8); r4 = _mm_andnot_pd(r8, r0); r5 = _mm_mul_pd(r1, _mm_set1_pd(0.37796447300922722721)); r6 = _mm_mul_pd(r1, _mm_set1_pd(0.24253562503633297352)); r7 = _mm_mul_pd(r1, _mm_set1_pd(4.1231056256176605498)); r8 = _mm_add_pd(r0, _mm_set1_pd(0.37796447300922722721)); r9 = _mm_add_pd(r1, _mm_set1_pd(0.24253562503633297352)); rA = _mm_sub_pd(r0, _mm_set1_pd(4.1231056256176605498)); rB = _mm_sub_pd(r1, _mm_set1_pd(4.1231056256176605498)); rC = _mm_set1_pd(1.4142135623730950488); rD = _mm_set1_pd(1.7320508075688772935); rE = _mm_set1_pd(0.57735026918962576451); rF = _mm_set1_pd(0.70710678118654752440); uint64 iMASK = 0x800fffffffffffffull; __m128d MASK = _mm_set1_pd(*(double*)&iMASK); __m128d vONE = _mm_set1_pd(1.0); uint64 c = 0; while (c < iterations) { size_t i = 0; while (i < 1000) { // Main computational loop r0 = _mm_mul_pd(r0, rC); r1 = _mm_add_pd(r1, rD); r2 = _mm_mul_pd(r2, rE); r3 = _mm_sub_pd(r3, rF); r4 = _mm_mul_pd(r4, rC); r5 = _mm_add_pd(r5, rD); r6 = _mm_mul_pd(r6, rE); r7 = _mm_sub_pd(r7, rF); r8 = _mm_mul_pd(r8, rC); r9 = _mm_add_pd(r9, rD); rA = _mm_mul_pd(rA, rE); rB = _mm_sub_pd(rB, rF); r0 = _mm_add_pd(r0, rF); r1 = _mm_mul_pd(r1, rE); r2 = _mm_sub_pd(r2, rD); r3 = _mm_mul_pd(r3, rC); r4 = _mm_add_pd(r4, rF); r5 = _mm_mul_pd(r5, rE); r6 = _mm_sub_pd(r6, rD); r7 = _mm_mul_pd(r7, rC); r8 = _mm_add_pd(r8, rF); r9 = _mm_mul_pd(r9, rE); rA = _mm_sub_pd(rA, rD); rB = _mm_mul_pd(rB, rC); r0 = _mm_mul_pd(r0, rC); r1 = _mm_add_pd(r1, rD); r2 = _mm_mul_pd(r2, rE); r3 = _mm_sub_pd(r3, rF); r4 = _mm_mul_pd(r4, rC); r5 = _mm_add_pd(r5, rD); r6 = _mm_mul_pd(r6, rE); r7 = _mm_sub_pd(r7, rF); r8 = _mm_mul_pd(r8, rC); r9 = _mm_add_pd(r9, rD);
The above is the detailed content of How to Achieve 4 FLOPs Per Cycle on Modern x86-64 Intel CPUs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


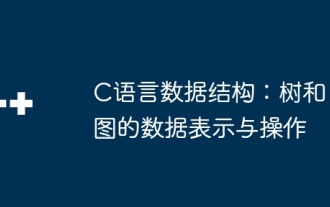
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
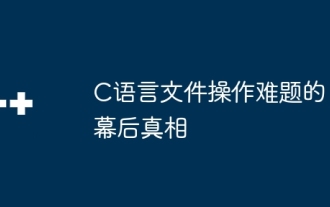
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
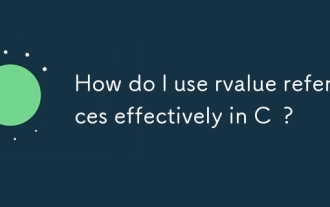
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
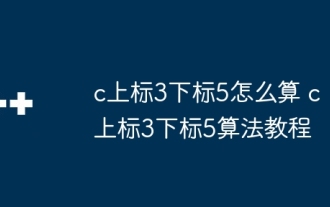
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
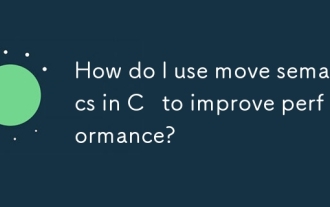
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
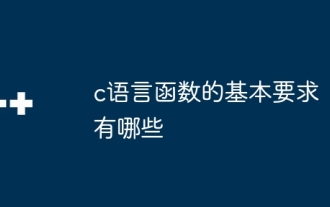
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
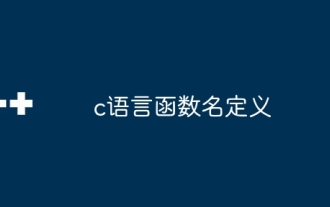
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
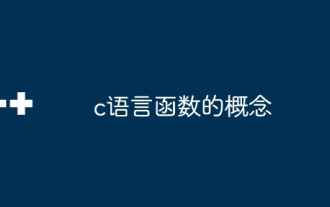
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
