How Can I Detect Firefox Versions 3 or 4 Using JavaScript?
Dec 14, 2024 pm 12:16 PMBrowser Version Detection
Problem:
How can you determine the version of a visitor's browser in JavaScript, specifically for Firefox versions 3 or 4?
Solution:
To detect a browser's version, rather than just its type, you can utilize JavaScript's navigator.userAgent property. This property contains a string with information about the browser, including the version number.
The following code snippet exemplifies a function that analyzes the navigator.userAgent string and extracts the browser version:
navigator.sayswho = (function () { var ua = navigator.userAgent; var tem; var M = ua.match(/(opera|chrome|safari|firefox|msie|trident(?=\/))\/?\s*(\d+)/i) || []; if (/trident/i.test(M[1])) { tem = /\brv[ :]+(\d+)/g.exec(ua) || []; return 'IE ' + (tem[1] || ''); } if (M[1] === 'Chrome') { tem = ua.match(/\b(OPR|Edge)\/(\d+)/); if (tem != null) return tem.slice(1).join(' ').replace('OPR', 'Opera'); } M = M[2] ? [M[1], M[2]] : [navigator.appName, navigator.appVersion, '-?']; if ((tem = ua.match(/version\/(\d+)/i)) != null) M.splice(1, 1, tem[1]); return M.join(' '); })(); console.log(navigator.sayswho); // outputs: `Chrome 62`
This function identifies and logs the browser version, which can be utilized for testing or logging purposes, enabling you to distinguish between different browser versions.
The above is the detailed content of How Can I Detect Firefox Versions 3 or 4 Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
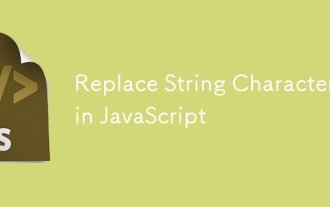
Replace String Characters in JavaScript
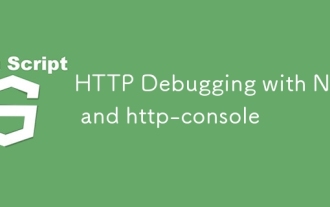
HTTP Debugging with Node and http-console
