


How Does `extern template` Avoid Redundant Template Instantiation in C ?
Dec 14, 2024 pm 12:50 PMUsing extern template to Avoid Template Instantiation (C 11)
In object-oriented programming, templates play a crucial role in providing generic solutions that work with different data types. However, when multiple source files instantiate templates with the same parameters, it can lead to multiple definitions and increased compile time.
The extern template keyword can be used to address this issue and avoid unnecessary template instantiation. It instructs the compiler not to instantiate a particular template specialization within the current translation unit. Instead, the compiler relies on the template being instantiated in another source file or linked library.
Function Templates
When dealing with function templates, extern template can be used to force exclusion of explicit template instantiation. For instance, consider the following code snippet:
// header.h template<typename T> void f(); // source1.cpp #include "header.h" void f<int>(); // Explicit instantiation // source2.cpp #include "header.h" extern template void f<int>(); // Prevents explicit instantiation void g() { f<int>(); }
In this scenario, source1.cpp explicitly instantiates f<int> while source2.cpp uses extern template to prevent unnecessary instantiation. The compiler will use the instantiation from source1.cpp when f<int> is called in source2.cpp.
Class Templates
The same principle applies to class templates. extern template can be used to avoid multiple instantiations of the same template class with the same parameters. Consider the following example:
// header.h template<typename T> class foo { public: void f(); }; // source1.cpp #include "header.h" template class foo<int>; // Explicit instantiation // source2.cpp #include "header.h" extern template class foo<int>; // Prevents explicit instantiation void h() { foo<int> obj; }
Here, extern template in source2.cpp prevents the explicit instantiation of foo<int>, ensuring that the instantiation from source1.cpp is used instead.
Benefits of Using extern template
Using extern template provides several benefits:
- Reduced compile time: By avoiding unnecessary template instantiations, compile time can be significantly reduced.
- Smaller object files: Multiple instantiations of the same template result in larger object files. extern template helps eliminate this problem.
- Consistent code: By ensuring that templates are instantiated only once, it promotes consistency and reduces the risk of errors.
Conclusion
extern template is a powerful keyword that allows developers to control template instantiation and optimize compile time. By using it judiciously, developers can ensure that templates are used efficiently and avoid the pitfalls of multiple instantiations, resulting in faster compilation and smaller executables.
The above is the detailed content of How Does `extern template` Avoid Redundant Template Instantiation in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
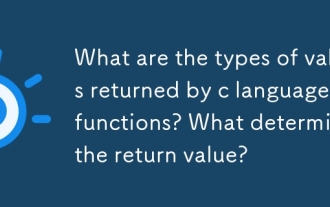
What are the types of values returned by c language functions? What determines the return value?
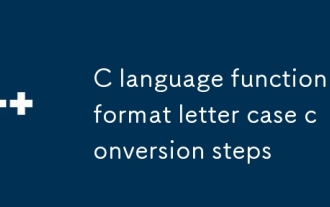
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
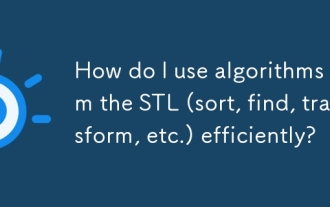
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
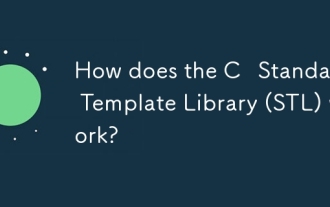
How does the C Standard Template Library (STL) work?
