Why Does Global Error Variable Initialization Fail in Go?
Dec 14, 2024 pm 02:51 PM<h2>Global Error Variable Initialization Failure</h2>
In a Go program, initializing an error variable globally poses a peculiar issue where it remains nil in other functions within the same package. To unravel this mystery, let's delve into a specific example:
<pre>package main
import (
"os" "fmt"
)
var loadErr error
func main() {
f, loadErr := os.Open("asdasd") if loadErr != nil { checkErr() } if f != nil { fmt.Println(f.Name()) }
}
// panic won't be called because loadErr is nil
func checkErr() {
if loadErr != nil { panic(loadErr) }
}
</pre>
In this scenario, you'd naturally expect the code to panic when the file cannot be opened. However, contrary to expectations, it remains silent due to the loadErr variable being nil. To resolve this, a crucial distinction must be made.
In Go, when using the := operator, a new local variable is created within the scope of the function. In this case, the line:
<pre>f, loadErr := os.Open("asdasd")</pre>
essentially constructs a local variable named loadErr, distinct from the globally declared variable. Unfortunately, the global variable remains unaffected, leading to the nil value conundrum.
To remedy this issue, the := operator must be replaced with the standard assignment operator =. This ensures that the global variable loadErr is referenced and initialized with the value returned by os.Open():
<pre>func main() {
_, loadErr = os.Open("asdasd")
</pre>
By making this subtle change, the global error variable is correctly set, and the panic function will now behave as intended.
Furthermore, if you require two return values from os.Open(), the second variable used in the assignment must be explicitly declared beforehand:
<pre>var f *os.File
f, loadErr = os.Open("asdasd")
</pre>
The above is the detailed content of Why Does Global Error Variable Initialization Fail in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
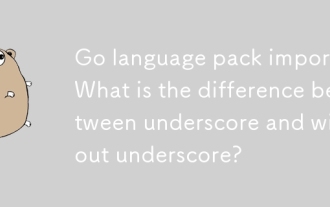
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
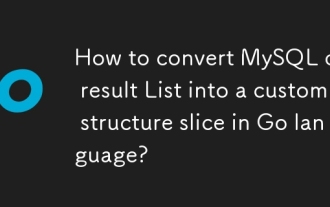
How to convert MySQL query result List into a custom structure slice in Go language?
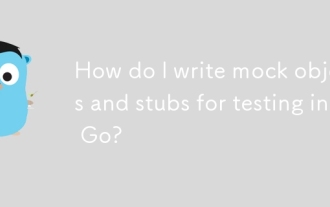
How do I write mock objects and stubs for testing in Go?
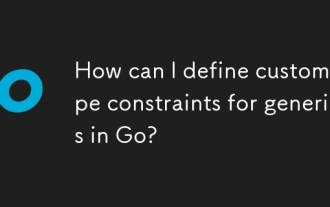
How can I define custom type constraints for generics in Go?
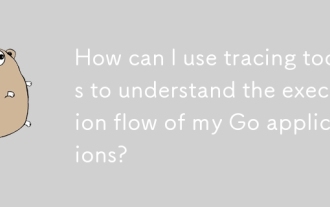
How can I use tracing tools to understand the execution flow of my Go applications?
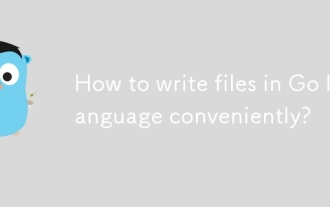
How to write files in Go language conveniently?
