


How to Create and Download a ZIP Archive of Multiple Files Using PHP?
Creating a ZIP Archive from Multiple Files in PHP
Question: How can I download multiple files as a ZIP archive using PHP?
Solution:
To achieve this, you can leverage PHP's ZipArchive class. Here's how you can proceed:
-
Create a ZIP Archive:
$files = array('readme.txt', 'test.html', 'image.gif'); $zipname = 'file.zip'; $zip = new ZipArchive; $zip->open($zipname, ZipArchive::CREATE);
Copy after login -
Add Files to the ZIP Archive:
foreach ($files as $file) { $zip->addFile($file); }
Copy after login -
Close the ZIP Archive:
$zip->close();
Copy after login -
Stream the ZIP Archive to the Client:
header('Content-Type: application/zip'); header('Content-disposition: attachment; filename="'.$zipname.'"'); header('Content-Length: ' . filesize($zipname)); readfile($zipname);
Copy after login
The first line in the streaming section specifies the Content-Type as a ZIP archive. The second line instructs the browser to display a download box for the user and assigns the filename file.zip. The third line calculates and sets the Content-Length header for older browsers that may encounter issues without knowing the file size in advance.
The above is the detailed content of How to Create and Download a ZIP Archive of Multiple Files Using PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


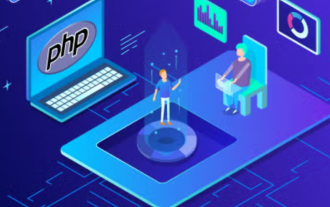
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
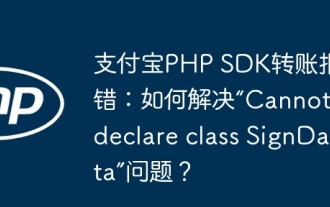
Alipay PHP...
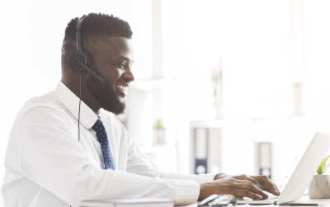
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
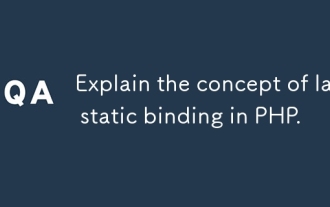
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
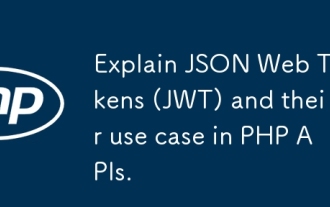
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
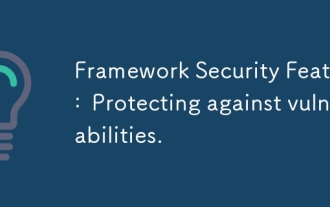
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
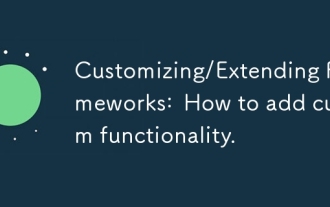
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
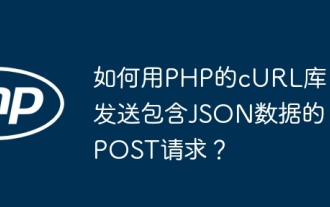
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
