How Can I Call Objective-C Methods from Within C Member Functions?
Calling Objective-C Methods from C Member Functions
Introduction:
Mixing C and Objective-C code can be essential for interoperability in iOS and macOS development. This article explores how to call Objective-C methods from within C member functions, addressing a common problem when working with cross-platform applications.
Objective-C Method Invocation from C :
The key to calling Objective-C methods from C lies in creating a C wrapper function. This function provides a C-style interface for the Objective-C method, making it accessible to C code.
C Wrapper Function:
Here's an example of a C wrapper function:
int MyObjectDoSomethingWith(void *myObjectInstance, void *parameter) { // Call the Objective-C method using Objective-C syntax return [(id)myObjectInstance doSomethingWith:parameter]; }
Objective-C Class Interface:
The Objective-C class that needs to be accessed from C should declare an interface that includes the target method:
@interface MyObject : NSObject { int someVar; } - (int)doSomethingWith:(void *)aParameter; @end
C Class Integration:
In the C class, you can include the header that declares the C wrapper function and use it to call the Objective-C method:
int MyCPPClass::someMethod(void *objectiveCObject, void *aParameter) { // Invoke the Objective-C method using the C trampoline function return MyObjectDoSomethingWith(objectiveCObject, aParameter); }
Alternative: PIMPL Implementation
The PIMPL (Pointer to Implementation) idiom can provide a more object-oriented approach by encapsulating the Objective-C instance inside a private implementation class:
// MyClassImpl.h (Private Implementation) class MyClassImpl { private: void *self; }; // MyObject (Objective-C) @interface MyObject : NSObject { int someVar; } - (void)logMyMessage:(char *)aCStr; @end // MyCPPClass (C++) class MyCPPClass { private: MyClassImpl * _impl; }; void MyCPPClass::doSomething() { _impl->logMyMessage("Hello from C++!"); }
Conclusion:
By following these techniques, you can effectively mix C and Objective-C code, allowing for seamless interoperability between the two languages in your iOS or macOS applications. Remember to consider the specific needs of your project and choose the approach that best suits your requirements.
The above is the detailed content of How Can I Call Objective-C Methods from Within C Member Functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










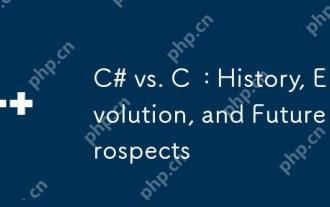
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
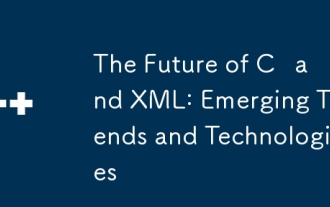
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
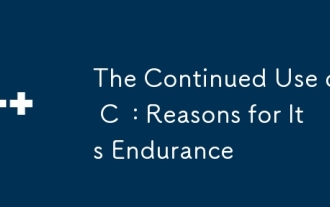
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
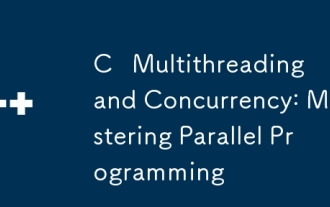
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
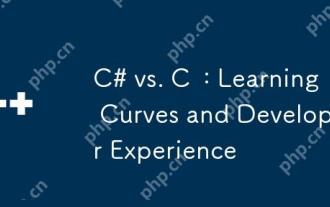
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
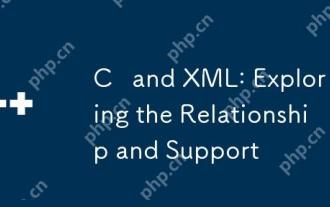
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
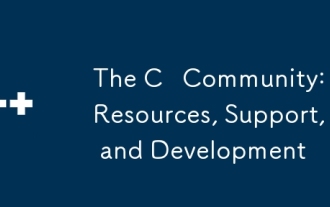
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
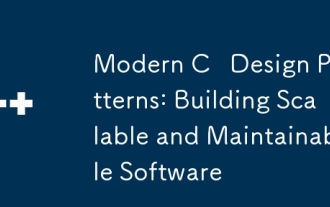
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
