


How to Position and Rotate Multiple Images in Golang with Proper Z-Index?
Image Manipulation in Golang
Query:
How do I position and rotate multiple images (i1 and i2) on a background image (bi) in Golang, ensuring the correct positioning and z-index for overlapping images?
Solution:
To achieve this, you can utilize the image package in Golang and leverage the graphics-go package for image manipulation. Here's a code snippet to visualize the solution:
package main import ( "fmt" "image" "image/draw" "image/jpeg" "code.google.com/p/graphics-go/graphics" "os" ) func main() { // Load the background image fImg1, _ := os.Open("background.jpg") defer fImg1.Close() bi, _, _ := image.Decode(fImg1) // Load the overlay images fImg2, _ := os.Open("overlay1.jpg") defer fImg2.Close() i1, _, _ := image.Decode(fImg2) fImg3, _ := os.Open("overlay2.jpg") defer fImg3.Close() i2, _, _ := image.Decode(fImg3) // Create a new image to hold the final result m := image.NewRGBA(image.Rect(0, 0, bi.Bounds().Max.X, bi.Bounds().Max.Y)) // Draw the background image draw.Draw(m, m.Bounds(), bi, image.Point{0, 0}, draw.Src) // Draw the overlay images with the specified positioning and rotation graphics.Rotate(m, i1, &graphics.RotateOptions{3.141592653589793, 0, 0}) graphics.Draw(m, m.Bounds(), i2, image.Point{100, 100}, draw.Src) // Save the final image to a file toimg, _ := os.Create("new.jpg") defer toimg.Close() jpeg.Encode(toimg, m, &jpeg.Options{jpeg.DefaultQuality}) fmt.Println("Image manipulation complete. Saved as 'new.jpg'.") }
In this example, the m variable represents the image canvas where the background and overlay images are drawn. The graphics.Rotate function is used to rotate the overlay images based on the provided z-index values. The final image is saved as "new.jpg" after the manipulation is complete.
The above is the detailed content of How to Position and Rotate Multiple Images in Golang with Proper Z-Index?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










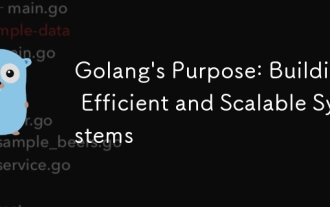
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
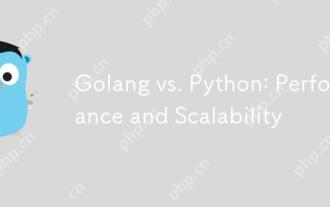
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
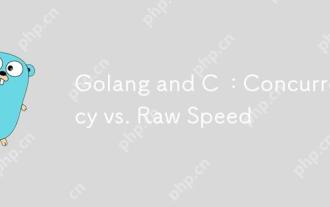
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
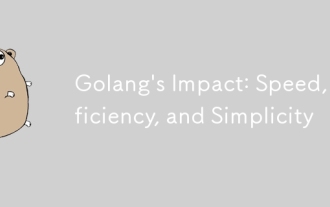
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
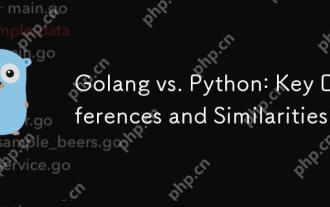
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
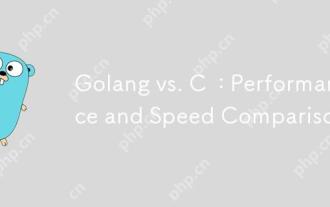
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
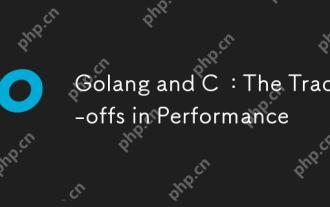
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
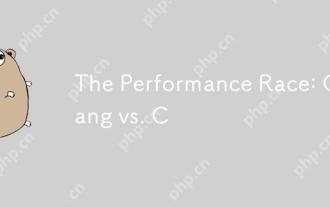
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
