How Can I Safely Return Local Arrays in C Without Warnings?
Dec 14, 2024 pm 05:45 PMReturning Local Arrays in C : Avoiding Warnings
Returning local arrays in C can trigger a warning like "returning address of local variable or temporary." To address this issue, consider an alternative approach that alleviates this warning:
Using std::vector<char>
In C , using std::vector<char> provides a dynamic alternative to local arrays. Here's an example:
std::vector<char> recvmsg() { std::vector<char> buffer(1024); //.. return buffer; } int main() { std::vector<char> reply = recvmsg(); }
By using std::vector<char>, we avoid the issue of returning a pointer to a local variable. The std::vector manages the memory for the char array automatically, eliminating the need to manually allocate and deallocate memory.
Accessing char* if Needed
If you still require a char* for C API compatibility, you can access it with &reply[0]. For instance:
void f(const char* data, size_t size) {} f(&reply[0], reply.size());
This allows you to use std::vector while still interfacing with C APIs that require char* parameters.
Benefits of Avoiding new
Employing std::vector avoids the use of new, which has the following benefits:
- No need for manual memory management
- Automatic memory deallocation when the std::vector goes out of scope
- Reduced risk of memory leaks
Conclusion
Using std::vector<char> is a more appropriate method for returning local arrays in C . It eliminates the warning associated with returning local variables while providing a dynamic memory management solution. For C API compatibility, &reply[0] can be used to access the char* representation of the std::vector.
The above is the detailed content of How Can I Safely Return Local Arrays in C Without Warnings?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
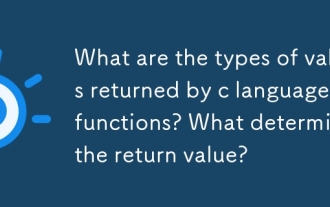
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
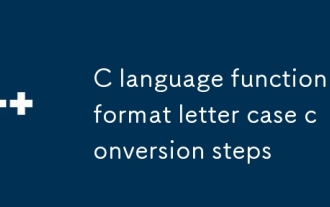
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
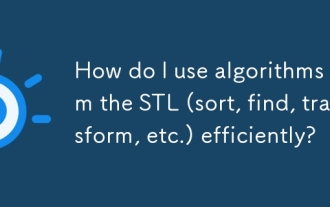
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
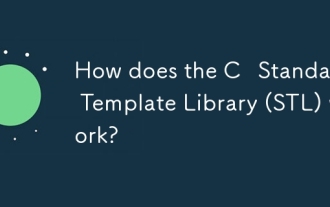
How does the C Standard Template Library (STL) work?
