How to Get a Filename Without Its Extension in Python?
Retrieving Filename without Extension from a Path in Python
Determining the filename excluding its extension is a common task when working with paths. In Python, achieving this can be accomplished in several ways.
Python 3.4 and Above
Leveraging the pathlib module's stem attribute provides a straightforward approach for retrieving the filename without the extension:
from pathlib import Path path = Path("/path/to/file.txt") filename_without_extension = path.stem print(filename_without_extension) # Outputs: "file"
Python Versions Prior to 3.4
For Python versions before 3.4, a combination of the os.path.splitext and os.path.basename functions can be utilized:
import os.path path = "/path/to/file.txt" filename = os.path.basename(path) filename_without_extension = os.path.splitext(filename)[0] print(filename_without_extension) # Outputs: "file"
This approach ensures compatibility with older Python versions while achieving the objective of extracting the filename without the extension.
The above is the detailed content of How to Get a Filename Without Its Extension in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


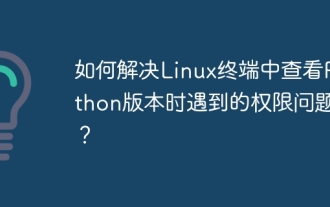
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
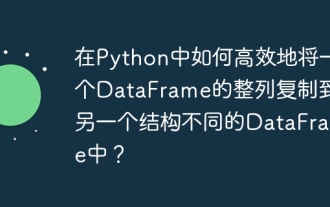
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
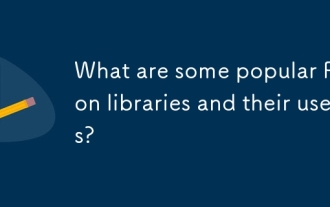
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
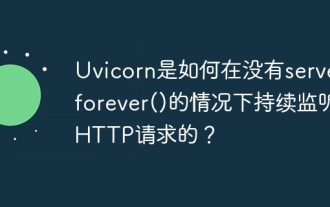
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
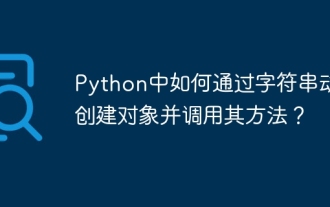
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
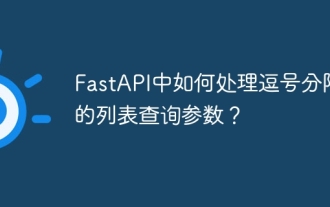
Fastapi ...
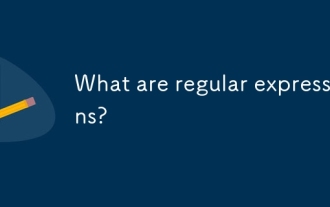
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
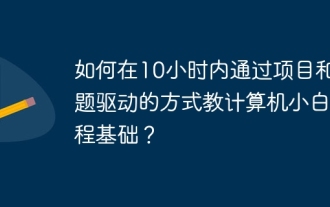
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
