How Can I Detect Invalid UTF-8 Byte Sequences in Go?
Detecting Invalid Byte Sequences in Go
In Go, when converting a byte slice ([]byte) to a string, it's possible to encounter invalid byte sequences that cannot be translated into Unicode. This arises from the fact that not all byte sequences represent valid UTF-8 characters.
To detect such occurrences, two approaches are available:
UTF-8 Validity Check:
As Tim Cooper mentions, the utf8.Valid function can be utilized to test if a byte slice contains valid UTF-8 bytes. If the result is false, it indicates the presence of invalid byte sequences.
String Conversion Considerations:
Contrary to common assumptions, Go permits the conversion of non-UTF-8 byte slices to strings. However, it's important to note that a string in Go is essentially a read-only byte slice and can therefore accommodate bytes that are not valid UTF-8.
It is only in specific situations that Go automatically performs UTF-8 decoding:
- When iterating over a string using the for i, r := range s syntax, the r variable represents a Unicode code point (rune) and is always valid.
- When converting from a string to a slice of runes (i.e., []rune(s)), Go decodes the entire string to runes.
In both cases, invalid UTF-8 characters are replaced with the U FFFD replacement character. This replacement may not be acceptable in all applications, so it's recommended to perform explicit UTF-8 validation if necessary.
Example:
Consider the following Go program:
package main import ( "fmt" "unicode/utf8" ) func main() { a := []byte{0xff} s := string(a) // Check UTF-8 validity if utf8.Valid(a) { fmt.Println("Valid UTF-8") } else { fmt.Println("Invalid UTF-8") } // Output string fmt.Println(s) }
Output:
Invalid UTF-8 �
In this example, the byte slice a contains an invalid byte sequence, resulting in an "Invalid UTF-8" message. Subsequently, when converting it to a string, the invalid byte is represented by the replacement character "�".
The above is the detailed content of How Can I Detect Invalid UTF-8 Byte Sequences in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










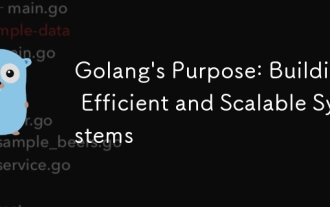
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
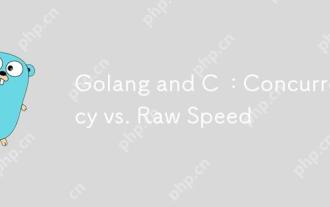
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
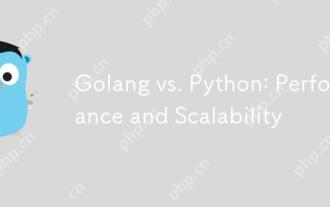
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
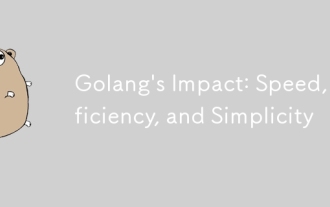
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
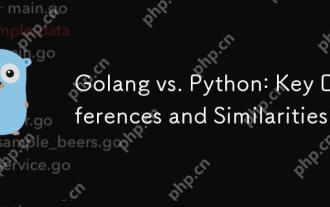
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
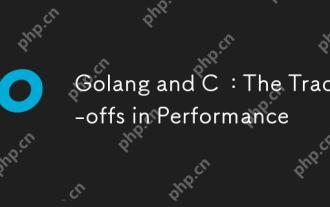
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
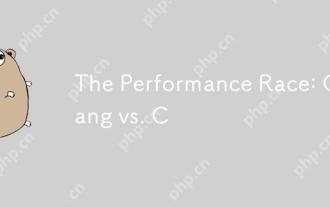
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
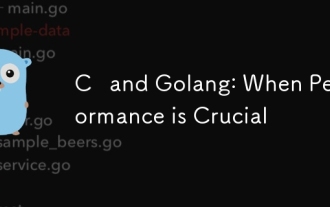
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
