How to Efficiently Retrieve DOM Elements by Class Name Using PHP?
How to Retrieve DOM Elements by Class Name
Retrieving sub-elements with specific class names from DOM nodes can be achieved using various methods. Here are some approaches:
Using PHP DOM
PHP DOM provides a powerful way to manipulate HTML documents. To grab elements with a given class name, you can use Xpath selectors:
$dom = new DomDocument(); $dom->load($filePath); $finder = new DomXPath($dom); $classname = "my-class"; $nodes = $finder->query("//*[contains(@class, '$classname')]");
Using Zend_Dom_Query
This library offers a convenient interface for working with CSS selectors, making it easier to select elements:
$finder = new Zend_Dom_Query($html); $classname = 'my-class'; $nodes = $finder->query("*[class~=\"$classname\"]");
Using Xpath Version of *[@class~='my-class'] CSS Selector
After further investigation, an Xpath version of the CSS selector was discovered:
[contains(concat(' ', normalize-space(@class), ' '), ' my-class ')]
Utilizing this xpath in PHP:
$finder = new DomXPath($dom); $classname = "my-class"; $nodes = $finder->query("//*[contains(concat(' ', normalize-space(@class), ' '), ' $classname ')]");
The above is the detailed content of How to Efficiently Retrieve DOM Elements by Class Name Using PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










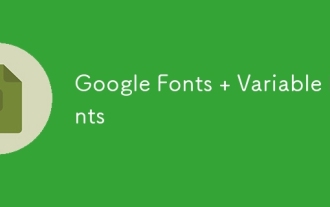
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
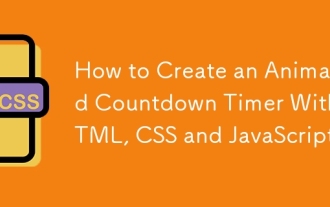
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
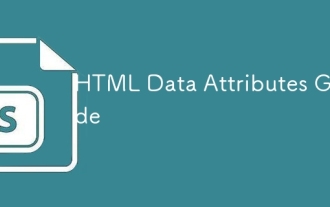
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
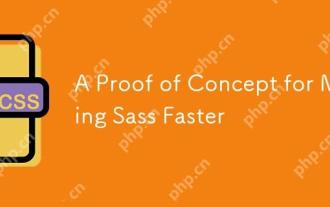
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
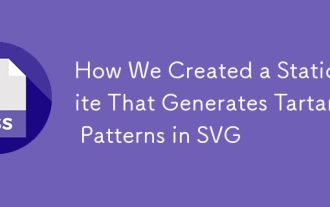
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
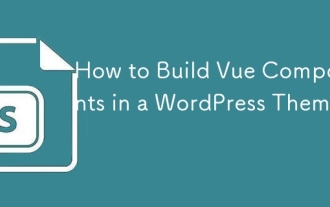
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
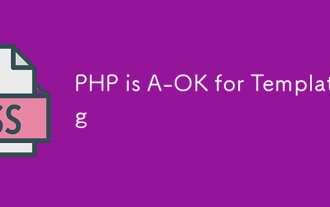
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
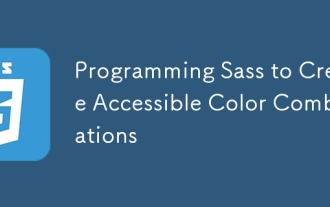
We are always looking to make the web more accessible. Color contrast is just math, so Sass can help cover edge cases that designers might have missed.
