


How Do Go Struct Methods Need to Match Interface Definitions to Avoid Type Mismatches?
Interface Implementation Restrictions on Struct Methods
In Go, a struct can implement an interface only if it has a method with the same name, type, and signature as the interface method. Consider the following code:
package main type A interface { Close() } type B interface { Connect() (A, error) } type C struct { /* ... */ } func (c *C) Close() {} type D struct { /* ... */ } func (d *D) Connect() (*C, error) { return &C{}, nil } // Return type mismatch func test(b B) {} func main() { d := &D{} test(d) // Error: type mismatch for Connect() method }
Here, the error message is: "cannot use d (type D) as type B in argument to test: D does not implement B (wrong type for Connect method)". This occurs because the return type of D's Connect method is *C, which does not match the (A, error) return type specified by the B interface.
Therefore, if a struct's method differs in its parameter or return type from the corresponding interface method, the struct does not implement the interface.
Resolving the Issue
To resolve this issue, the Connect method of struct D must align with the Connect method of interface B. This involves ensuring that it returns the expected (A, error) type.
import "fmt" type A interface { Close() } type B interface { Connect() (A, error) } type C struct { name string } func (c *C) Close() { fmt.Println("C closed") } type D struct {} func (d *D) Connect() (A, error) { return &C{"D"}, nil } func test(b B) { b.Connect().Close() // Call Close() on the returned A } func main() { test(&D{}) }
With this modification, the code compiles and runs without errors, as the Connect method of struct D now adheres to the B interface's definition.
The above is the detailed content of How Do Go Struct Methods Need to Match Interface Definitions to Avoid Type Mismatches?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










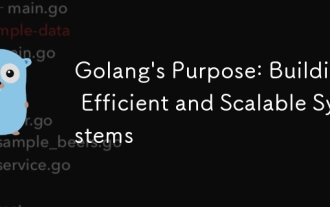
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
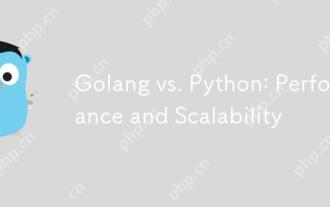
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
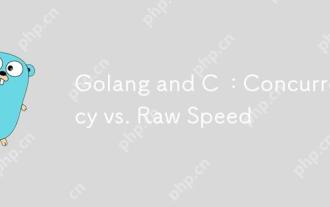
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
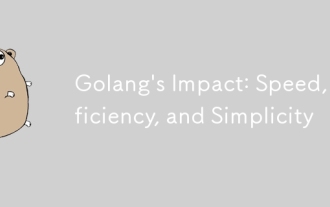
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
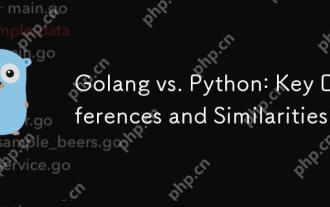
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
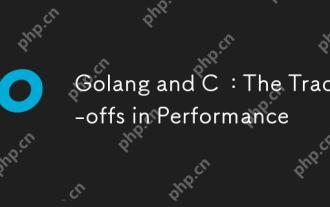
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
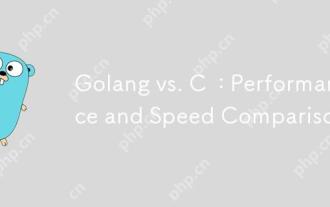
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
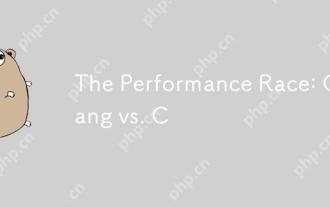
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
