How to Elegantly Update Objects within Arrays in ReactJS?
Elegant Object Updates in Arrays for ReactJS
In ReactJS, managing arrays of objects within your application's state can be a common scenario. One such task is updating a specific object within an array, which can pose a challenge without a clear understanding of the available techniques.
Example Scenario
Consider the following React component example:
var CommentBox = React.createClass({ getInitialState: function() { return { data: [ { id: 1, author: "john", text: "foo" }, { id: 2, author: "bob", text: "bar" }, ], }; }, handleCommentEdit: function(id, text) { // Handle updating of the object inside the array }, });
The objective is to update the object in this.state.data when the handleCommentEdit method is called, specifically modifying the text property.
Object.assign() Approach
A commonly preferred method is using Object.assign(). It offers a concise way to update an existing object without creating a new one.
handleCommentEdit: function(id, text) { this.setState({ data: this.state.data.map(el => (el.id === id ? Object.assign({}, el, { text }) : el)), }); }
Here, we iterate through each element in this.state.data. If an element's id matches the provided id, we create a new object by combining the original el with the updated text using Object.assign(). Otherwise, we return the original element.
Spread Operator Approach
For a more modern approach, you can utilize the ES2018 spread operator:
handleCommentEdit: function(id, text) { this.setState({ data: this.state.data.map(el => (el.id === id ? { ...el, text } : el)), }); }
Similar to the Object.assign() method, we use the spread operator to create a new object, combining the original object with the updated text property.
Both the Object.assign() and spread operator approaches provide an efficient and elegant way to update objects within an array while maintaining immutability in ReactJS applications.
The above is the detailed content of How to Elegantly Update Objects within Arrays in ReactJS?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
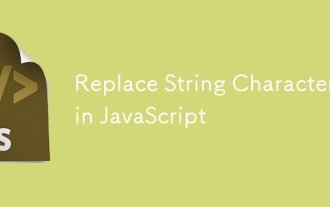
Replace String Characters in JavaScript
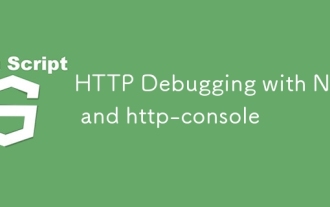
HTTP Debugging with Node and http-console
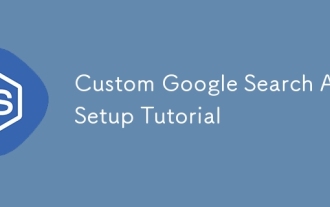
Custom Google Search API Setup Tutorial
