


How Can I Consistently Retrieve File Creation and Modification Dates Across Linux and Windows?
Cross-Platform Retrieval of File Creation and Modification Dates
Determining file creation and modification dates/times consistently across platforms has been a persistent challenge. Here's a comprehensive breakdown of the best approaches for Linux and Windows:
Getting File Modification Dates
Retrieving the last modified timestamp is straightforward in both Linux and Windows. Simply use the os.path.getmtime(path) function. It returns the Unix timestamp of the most recent modification to the file at the specified path.
Getting File Creation Dates
Extracting file creation dates, however, proves more complex and platform-dependent:
-
Windows:
Windows maintains a creation date (ctime) for files. Access this information through os.path.getctime(path) or the .st_ctime attribute of the result from os.stat(). -
Mac:
MacOS and certain Unix-based systems provide a .st_birthtime attribute that stores the file's creation date. -
Linux:
Currently, determining file creation dates on Linux is not possible without writing a C extension for Python. However, the Linux kernel returns the file's last modified timestamp through st_mtime, which can serve as a reasonable proxy.
Cross-Platform Compatibility
For cross-platform compatibility, consider the following code:
import os import platform def creation_date(path_to_file): """ Retrieve the date the file was created. If not possible, fall back to the last modified date. """ if platform.system() == 'Windows': return os.path.getctime(path_to_file) else: stat = os.stat(path_to_file) try: return stat.st_birthtime except AttributeError: # Assuming Linux, fall back to modification date return stat.st_mtime
By leveraging platform-specific techniques and handling exceptions appropriately, this code allows for consistent retrieval of file creation and modification dates on both Linux and Windows.
The above is the detailed content of How Can I Consistently Retrieve File Creation and Modification Dates Across Linux and Windows?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










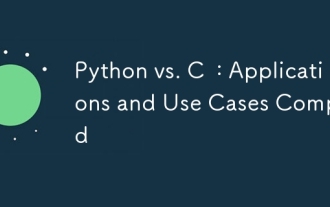
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
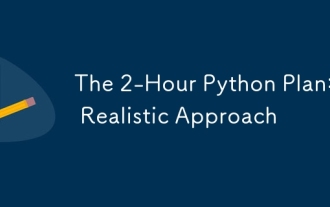
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
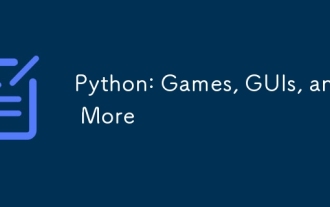
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
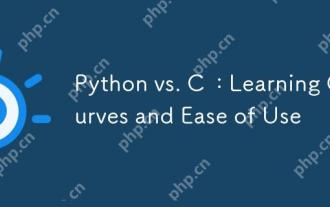
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
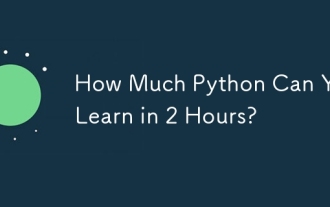
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
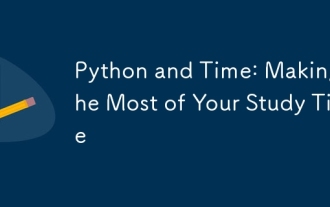
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
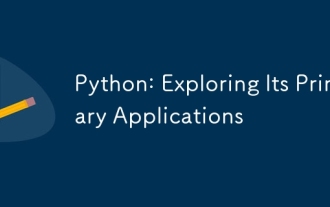
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
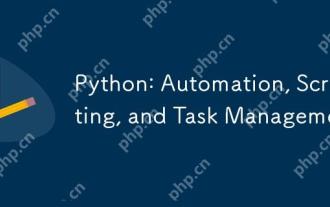
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
