


How to Concatenate Strings from Multiple Rows in Pandas Using Groupby?
Concatenate Strings from Multiple Rows using Pandas Groupby
When working with dataframes, there may be situations where you need to consolidate strings from multiple rows while grouping them by specific criteria. Pandas offers a convenient solution for this through its groupby and transform functions.
Problem Statement
Given a dataframe with columns 'name,' 'text,' and 'month,' the goal is to concatenate the strings in the 'text' column for each unique combination of 'name' and 'month.' The desired output is a dataframe with unique 'name' and 'month' combinations and the concatenated 'text' values.
Solution
To achieve this, you can utilize the following steps:
- Group the dataframe by 'name' and 'month' using the groupby() function.
- Use the transform() function to apply a lambda expression that joins the 'text' entries for each group.
- To remove duplicate rows, drop the duplicates from the resulting dataframe using the drop_duplicates() function.
Here's an example code:
import pandas as pd from io import StringIO data = StringIO(""" "name1","hej","2014-11-01" "name1","du","2014-11-02" "name1","aj","2014-12-01" "name1","oj","2014-12-02" "name2","fin","2014-11-01" "name2","katt","2014-11-02" "name2","mycket","2014-12-01" "name2","lite","2014-12-01" """) # load string as stream into dataframe df = pd.read_csv(data, header=0, names=["name", "text", "date"], parse_dates=[2]) # add column with month df["month"] = df["date"].apply(lambda x: x.month) df['text'] = df[['name','text','month']].groupby(['name','month'])['text'].transform(lambda x: ','.join(x)) df[['name','text','month']].drop_duplicates()
The above code generates a dataframe with the desired result:
name text month 0 name1 hej,du 11 2 name1 aj,oj 12 4 name2 fin,katt 11 6 name2 mycket,lite 12
Alternative Solution
Instead of using transform(), you can also utilize apply() and then reset_index() to achieve the same result. The updated code would be:
df.groupby(['name','month'])['text'].apply(','.join).reset_index()
This simplified version eliminates the lambda expression and provides a more concise solution.
The above is the detailed content of How to Concatenate Strings from Multiple Rows in Pandas Using Groupby?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










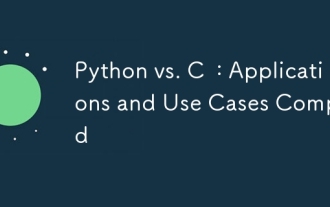
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
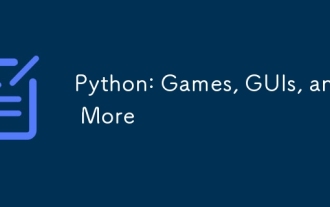
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
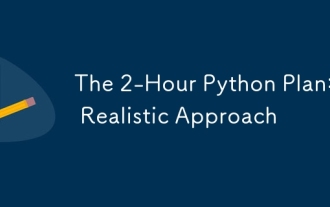
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
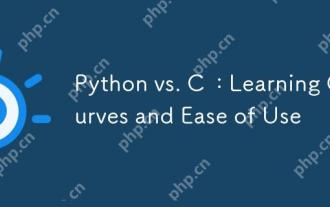
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
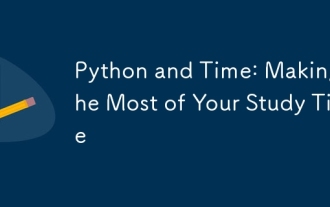
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
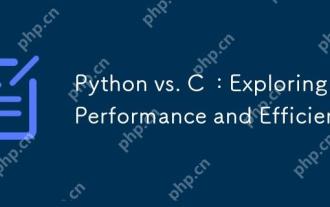
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
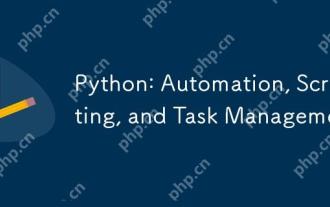
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
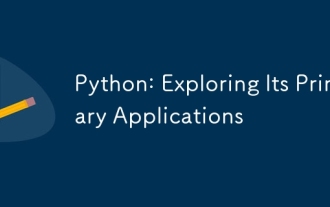
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
