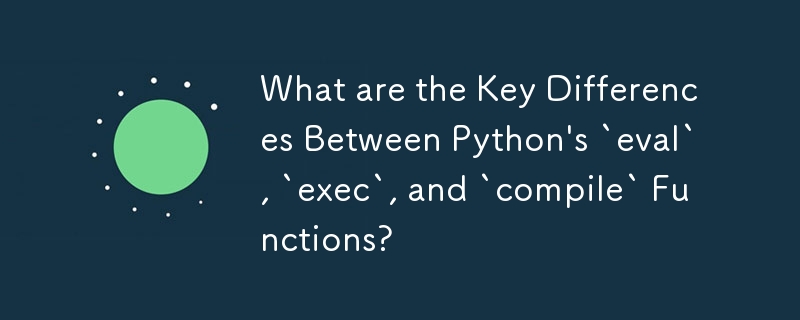
Understanding the Differences Between eval, exec, and compile
Introduction
In Python, eval, exec, and compile are powerful tools for dynamic code evaluation and execution. However, their distinct functionalities and modes of operation can be confusing. This article clarifies the key differences between these functions, highlighting their unique roles in handling dynamic code.
eval vs. exec
eval:
- Evaluates a single Python expression and returns its value.
- Accepts a string containing a valid Python expression, such as '42 1' or 'x * 5'.
exec:
- Executes a Python code block for its side effects, such as statements, loops, or function/class definitions.
- Accepts a string containing a complete Python code block, which can include multiple statements.
Key Distinctions:
-
Scope and Result: eval returns the value of the evaluated expression, while exec ignores the return value and always returns None.
-
Code Block: eval works with expressions only, while exec can handle entire code blocks with statements.
Compile Modes
The compile function provides a way to compile source code into bytecode before execution. It has three modes:
'eval':
- Compiles a single expression into bytecode that returns its value.
'exec':
- Compiles multiple statements into bytecode that always returns None.
'single':
- A limited form of 'exec' that compiles a single statement (or multiple statements if the last is an expression), printing the expression's value to the standard output.
When to Use Each Function
- Use eval when you need to evaluate a dynamically generated expression and retrieve its result (e.g., calculate a value based on user input).
- Use exec when you want to execute a code block for its side effects, such as modifying global variables or performing calculations.
- Use compile to pre-compile source code into bytecode for improved execution speed when handling repetitive code.
Code Examples
# Eval: Evaluate an expression
result = eval('42 + 1') # Returns 43
# Exec: Execute code for side effects
exec('print("Hello, world!")') # Prints "Hello, world!"
# Compile and eval: Pre-compile, then evaluate
code = compile('x + 1', '<string>', 'eval')
result = eval(code) # Assumes 'x' is defined in the global scope
Copy after login
Conclusion
eval, exec, and compile are powerful tools for dynamic code handling. Understanding their differences is crucial for effectively utilizing them in your Python projects. By choosing the appropriate function and mode, you can optimize code execution, enhance code flexibility, and unlock the full potential of dynamic code evaluation in Python.
The above is the detailed content of What are the Key Differences Between Python's `eval`, `exec`, and `compile` Functions?. For more information, please follow other related articles on the PHP Chinese website!