


What's the Most Efficient Way to Access the First Element of a PHP Array?
Dec 15, 2024 pm 01:18 PMAccessing Array Elements Efficiently
When working with arrays in PHP, retrieving the first element is a common task. This operation can be performed in various ways, each with its own performance implications.
Original Option:
The original suggestion of using array_shift(array_values($array)), while straightforward, is computationally expensive due to the need to create a new copy of the array.
O(1) Solution:
A more efficient and constant-time (O(1)) approach involves reversing the array and popping the last element:
array_pop(array_reverse($array));
This operation avoids the need for a copy and directly extracts the first element.
Alternative Strategies:
If modifying the original array is acceptable, reset($array) can be used to set the internal array pointer to the first element.
For cases requiring an array copy, consider array_shift(array_slice($array, 0, 1)).
In PHP 5.4 , the compact array_values($array)[0] syntax can also be employed, but it may raise an error for empty arrays.
The above is the detailed content of What's the Most Efficient Way to Access the First Element of a PHP Array?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
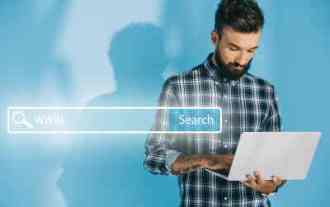
11 Best PHP URL Shortener Scripts (Free and Premium)
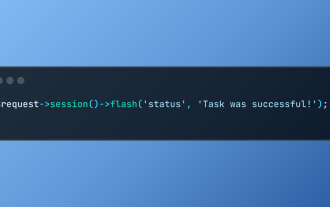
Working with Flash Session Data in Laravel
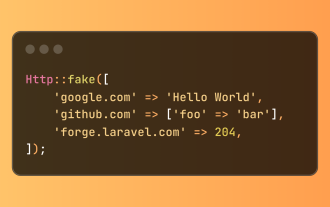
Simplified HTTP Response Mocking in Laravel Tests
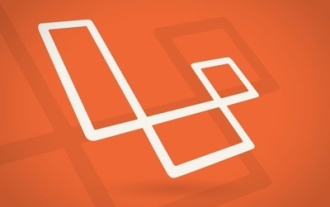
Build a React App With a Laravel Back End: Part 2, React
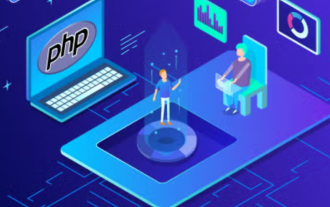
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
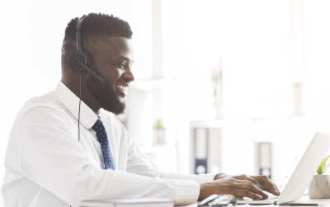
12 Best PHP Chat Scripts on CodeCanyon
