


How Can I Effectively Handle JSON Marshaling and Unmarshaling with Go's Interface Types?
Marshaling to Interface Types
JSON marshaling and unmarshaling can present challenges when working with interface types. This article delves into the difficulties and provides solutions.
Marshaling from Interface
Marshaling from an interface type is straightforward because the underlying type is known locally, allowing the reflector to identify it.
Unmarshaling to Interface
Unmarshaling to an interface type, however, is problematic. Without knowing the concrete type, the reflector cannot instantiate a new instance to receive the marshaled data.
Resolving the Issue
To address this limitation, one approach is to implement the Unmarshaler interface for custom types. Refer to the example below:
import ( "encoding/json" "fmt" "log" ) // RawString represents a raw JSON object. type RawString string // Implement the Marshaler and Unmarshaler interfaces. func (m *RawString) MarshalJSON() ([]byte, error) { return []byte(*m), nil } func (m *RawString) UnmarshalJSON(data []byte) error { *m += RawString(data); return nil } // Example data. const data = `{"i":3, "S":{"phone": {"sales": "2223334444"}}}` // Example struct. type A struct { I int64 S RawString `sql:"type:json"` } func main() { a := A{} if err := json.Unmarshal([]byte(data), &a); err != nil { log.Fatal("Unmarshal failed:", err) } fmt.Println("Done:", a) }
In this example, RawString implements both Marshaler and Unmarshaler, allowing for custom marshaling and unmarshaling. The main function then uses json.Unmarshal to decode the JSON data into an instance of A.
The above is the detailed content of How Can I Effectively Handle JSON Marshaling and Unmarshaling with Go's Interface Types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










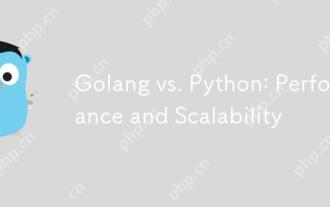
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
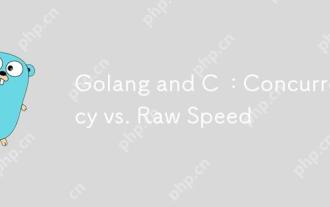
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
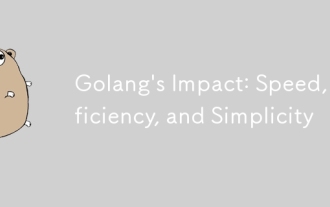
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
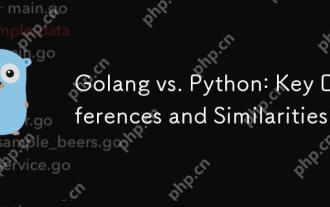
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
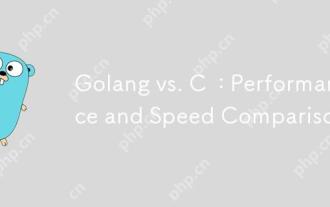
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
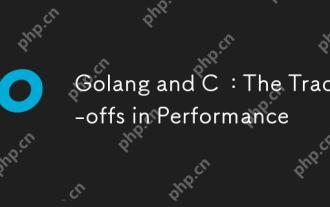
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
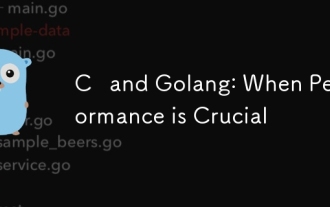
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
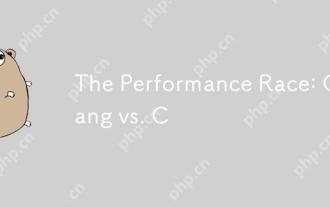
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
