


How Do I Correctly Append Multiple Byte Slices in Go Using `append`?
Dec 15, 2024 pm 08:35 PMAppending Binary Slices with Variable Arguments
You've encountered an error while trying to append two byte slices (one and two) using append. The issue stems from attempting to pass both slices as []byte without using the correct syntax for passing variable arguments in Go.
As per the Go Programming Language Specification:
"append(s S, x ...T) S // T is the element type of S"
For your case, where the final argument (two) is a []byte slice, you must append the ... operator:
"If the final argument is assignable to a slice type []T, it may be passed unchanged as the value for a ...T parameter if the argument is followed by ...."
Therefore, the correct code is:
import "fmt" func main() { one := make([]byte, 2) two := make([]byte, 2) fmt.Println(append(one[:], two[:]...)) }
This appends the two slices correctly and prints the combined binary data.
The above is the detailed content of How Do I Correctly Append Multiple Byte Slices in Go Using `append`?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
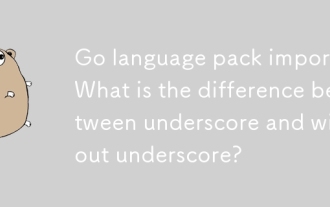
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
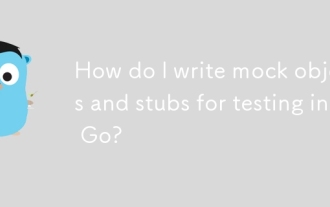
How do I write mock objects and stubs for testing in Go?
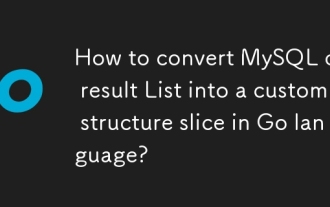
How to convert MySQL query result List into a custom structure slice in Go language?
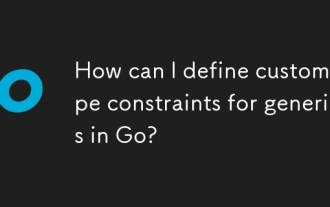
How can I define custom type constraints for generics in Go?
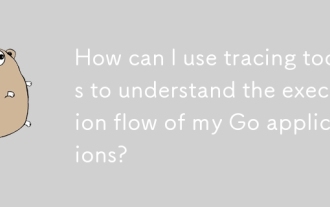
How can I use tracing tools to understand the execution flow of my Go applications?
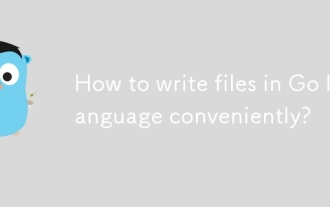
How to write files in Go language conveniently?
