


How Can I Find All Indices of a Specific Element in a Python List?
Obtaining All Occurrences of an Element in a List
While Python's index() method provides the index of an element's first occurrence, this article explores an efficient trick to retrieve all its occurrences in a list.
The Solution: List Comprehension with Enumeration
To accomplish this, we employ a list comprehension along with the enumerate() function:
indices = [i for i, x in enumerate(my_list) if x == "whatever"]
Understanding the Implementation
The enumerate(my_list) function generates pairs of the form (index, item) for every item in the list. Subsequently, the list comprehension retrieves the index i and the list item x from these pairs.
By applying the condition x == "whatever", we filter the pairs to only include those where x matches our desired value. The resulting list indices contains the indices of all occurrences of "whatever" in the original list.
This technique is especially useful when you need to perform subsequent operations on multiple occurrences of a particular element within a list, without iterating over it.
The above is the detailed content of How Can I Find All Indices of a Specific Element in a Python List?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
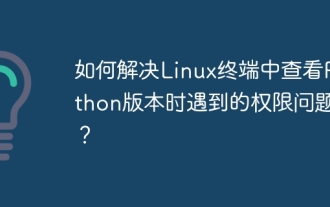
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
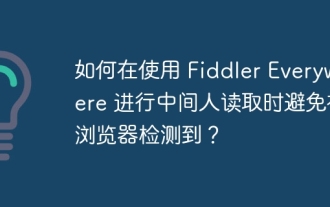
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
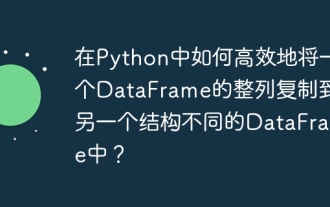
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
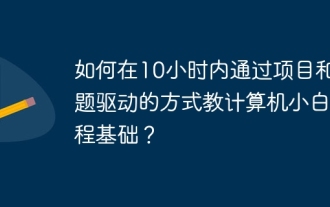
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
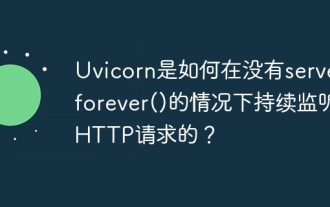
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
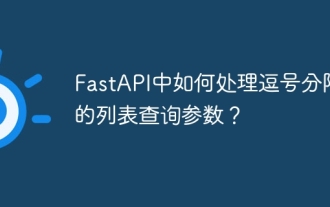
Fastapi ...
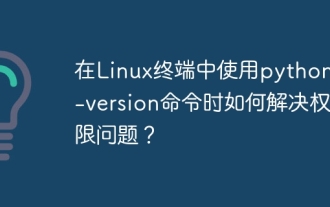
Using python in Linux terminal...
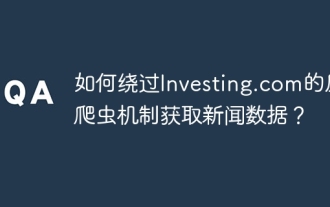
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
