How Can I Transform Black to Any RGB Color Using Only CSS Filters?
Recoloring Black into Any Color Using Only CSS Filters
Problem:
Given a target RGB color, transform black (#000) into that color using only CSS filters.
Solution:
The solution involves a function that takes a target color as input and returns a CSS filter string that transforms black into the target color.
The function uses a combination of filters, including invert, sepia, saturate, hue-rotate, brightness, and contrast, to achieve the desired effect.
- Invert: Inverts the colors, turning black into white.
- Sepia: Adds a sepia tint, warming the color.
- Saturate: Adjusts the saturation of the color.
- Hue-Rotate: Rotates the hue of the color.
- Brightness: Adjusts the brightness of the color.
- Contrast: Adjusts the contrast of the color.
Implementation:
function recolorBlack(targetColor) { // Convert RGB color to HSL const hsl = targetColor.toHSL(); // Calculate filter values const invert = (255 - targetColor.r) / 255; const sepia = (targetColor.g - targetColor.b) / 255; const saturate = targetColor.s / 100; const hueRotate = hsl.h; const brightness = targetColor.l / 100; const contrast = (targetColor.r - targetColor.g + targetColor.b - 128) / 128; // Generate CSS filter string return `filter: invert(${invert * 100}%) sepia(${sepia * 100}%) saturate(${saturate * 100}%) hue-rotate(${hueRotate}deg) brightness(${brightness * 100}%) contrast(${contrast * 100}%);`; }
Example:
.element { background-color: black; filter: recolorBlack(rgb(255, 0, 0)); }
This will apply a blue tint to the element because blue is specified as the 'rgb' parameter ((255, 0, 0) is blue).
Note: The above JavaScript function and CSS implementation are hypothetical for illustrative purposes. The actual implementation may vary.
The above is the detailed content of How Can I Transform Black to Any RGB Color Using Only CSS Filters?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










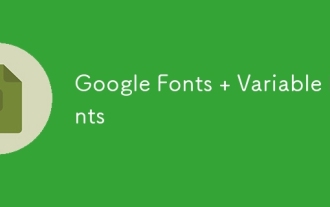
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
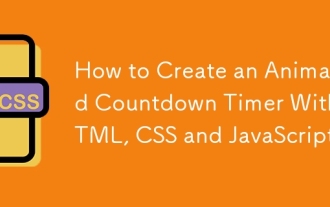
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
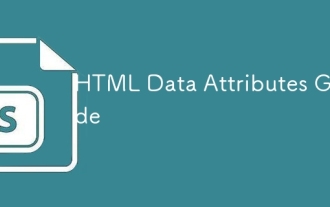
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
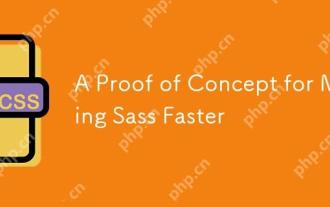
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
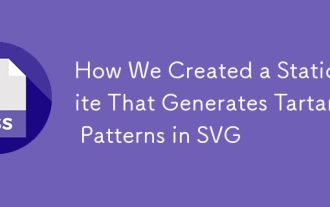
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
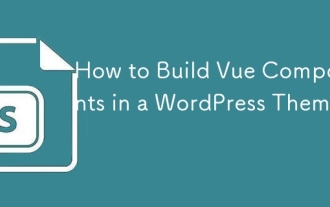
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
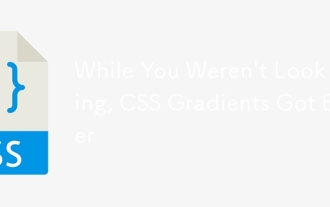
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
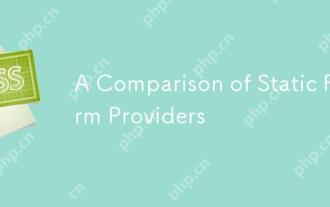
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
