React & Expo - How to Upload & Download Files
Introduction
I struggled to find clear examples on how to upload and download files in an Expo-based mobile app. To help others facing the same challenge—or anyone simply curious—I wrote this post.
Along the way, we’ll explore key concepts that are valuable to understand:
- Buffers
- Intent Filters
- MIME types
- application/octet-stream
- multipart/form-data
- And more...
What we’ll cover:
- Sending and receiving files with a Fastify server.
- Uploading, downloading, and displaying files on a React web app.
- Uploading, downloading, and displaying files on a React Native (Expo) mobile app.
All code and the Postman collection are available in my GitHub.
Server
The server runs on Fastify (a modernized version of Express.js). To start the app do the fallowing:
- Using the terminal navigate to /server
- Install the dependencies with npm install
- Run the server using npm run dev
In app.js we have three key endpoints:
- Download endpoint (/download)
fastify.get("/download", async function handler(_, reply) { const fd = await open(FILE_TO_DOWNLOAD); const stream = fd.createReadStream(); const mimeType = mime.lookup(FILE_TO_DOWNLOAD); console.log(`Downloading -> ${FILE_TO_DOWNLOAD}`); return reply .type(mimeType) .header( "Content-Disposition", `attachment; filename=${path.basename(FILE_TO_DOWNLOAD)}` ) .send(stream); });
This endpoint sends example.webp as a stream using createReadStream(). The MIME type is included so the client knows how to handle the file. For example.webp, this will be image/webp.
?Note: The MIME type defines the format of the file being sent. This helps the client display it correctly.
See more MIME types.
The Content-Disposition header defines how the content should be presented to the client. Including attachment; filename=
Learn more about Content-Disposition
- Upload Multiples files using Form Data (/upload-multiples)
fastify.post("/upload-multiples", async function handler(request) { const parts = request.files(); const uploadResults = []; for await (const file of parts) { const fileBuffer = await file.toBuffer(); const filename = file.filename; const filePath = path.join(DIR_TO_UPLOAD, filename); await writeFile(filePath, fileBuffer); uploadResults.push({ filename, uploaded: true }); console.log(`Uploaded -> ${filePath}`); } return { uploadedFiles: uploadResults }; });
This endpoint accepts a multipart/form-data request. It:
- Retrieves the files from the request.
- Converts each file to a Buffer (a JavaScript representation of binary data).
- Saves the file to the /upload directory.
For example, a request might look like this:
- Upload Files using Octet Stream (/upload-octet-stream)
fastify.get("/download", async function handler(_, reply) { const fd = await open(FILE_TO_DOWNLOAD); const stream = fd.createReadStream(); const mimeType = mime.lookup(FILE_TO_DOWNLOAD); console.log(`Downloading -> ${FILE_TO_DOWNLOAD}`); return reply .type(mimeType) .header( "Content-Disposition", `attachment; filename=${path.basename(FILE_TO_DOWNLOAD)}` ) .send(stream); });
This endpoint expects a single binary file in the request body (application/octet-stream). Unlike multipart/form-data, the file is already binary data, so we can write it directly to disk.
The request will look something like this in Postman:
Web (React)
To run the app:
- Using the terminal navigate to /web
- Install the dependencies with npm install
- Start the app using npm run dev
All functionality for the web application is contained in App.tsx:
This React app provides three key features:
- Download/Display File
fastify.post("/upload-multiples", async function handler(request) { const parts = request.files(); const uploadResults = []; for await (const file of parts) { const fileBuffer = await file.toBuffer(); const filename = file.filename; const filePath = path.join(DIR_TO_UPLOAD, filename); await writeFile(filePath, fileBuffer); uploadResults.push({ filename, uploaded: true }); console.log(`Uploaded -> ${filePath}`); } return { uploadedFiles: uploadResults }; });
When the user clicks the "Download" button, the app:
- Calls the /download endpoint.
- Receives the file as a binary blob.
- Creates an objectURL from the blob, acting as a temporary URL that the browser can access.
The behavior depends on the Content-Disposition header returned by the server:
- If Content-Disposition includes inline, the file is displayed in a new tab.
- If it includes attachment, the file is downloaded automatically.
To trigger the download, the app creates a temporary element with the href set to the objectURL and programmatically clicks it, simulating a user download action.
- Upload File using Form Data
fastify.post("/upload-octet-stream", async function handler(request) { const filename = request.headers["x-file-name"] ?? "unknown.text"; const data = request.body; const filePath = path.join(DIR_TO_UPLOAD, filename); await writeFile(filePath, data); return { uploaded: true }; });
When the "Upload File" button is clicked:
- The uploadFile function runs, creating a hidden element and simulating a user click.
- Once the user selects one or more files, those files are appended to a FormData object.
- The request is sent to the /upload-multiples endpoint, which accepts files via multipart/form-data.
This allows the server to properly handle and save the uploaded files.
- Upload Files using Octet Stream
const downloadFile = async () => { const response = await fetch(DOWNLOAD_API); if (!response.ok) throw new Error("Failed to download file"); const blob = await response.blob(); const contentDisposition = response.headers.get("Content-Disposition"); const isInline = contentDisposition?.split(";")[0] === "inline"; const filename = contentDisposition?.split("filename=")[1]; const url = window.URL.createObjectURL(blob); if (isInline) { window.open(url, "_blank"); } else { const a = document.createElement("a"); a.href = url; a.download = filename || "file.txt"; a.click(); } window.URL.revokeObjectURL(url); };
This approach is simpler than using multipart/form-data—just send the file directly in the request body as binary data, and include the filename in the request headers.
Mobile (Expo)
You can start the app with the fallowing:
- Navigate to the mobile directory in your terminal.
- Install the dependencies: npm install
- Run the proyect with npm run android or npm run ios
The main logic is in App.tsx where it renders the fallowing:
fastify.get("/download", async function handler(_, reply) { const fd = await open(FILE_TO_DOWNLOAD); const stream = fd.createReadStream(); const mimeType = mime.lookup(FILE_TO_DOWNLOAD); console.log(`Downloading -> ${FILE_TO_DOWNLOAD}`); return reply .type(mimeType) .header( "Content-Disposition", `attachment; filename=${path.basename(FILE_TO_DOWNLOAD)}` ) .send(stream); });
To display the file in a new view (Like when the browser open the file in a new tab) we have to read the response as a blob and then transform it to base64 using FileReader.
We write the file in the cache directory (a private directory where only the app have access) and then display it using IntentLauncher or Sharing if the user is using iOS.
- Download File
fastify.post("/upload-multiples", async function handler(request) { const parts = request.files(); const uploadResults = []; for await (const file of parts) { const fileBuffer = await file.toBuffer(); const filename = file.filename; const filePath = path.join(DIR_TO_UPLOAD, filename); await writeFile(filePath, fileBuffer); uploadResults.push({ filename, uploaded: true }); console.log(`Uploaded -> ${filePath}`); } return { uploadedFiles: uploadResults }; });
This is similar to the web process but we have to read the blob as a base64 using FileReader and then ask for permission to donwload the file where the user wants to save the file.
- Upload File using Form Data
fastify.post("/upload-octet-stream", async function handler(request) { const filename = request.headers["x-file-name"] ?? "unknown.text"; const data = request.body; const filePath = path.join(DIR_TO_UPLOAD, filename); await writeFile(filePath, data); return { uploaded: true }; });
Use DocumentPicker to enable users to select files, then utilize FormData to append the selected files to the request. The process is very straightforward.
- Upload File as Octet Stream
const downloadFile = async () => { const response = await fetch(DOWNLOAD_API); if (!response.ok) throw new Error("Failed to download file"); const blob = await response.blob(); const contentDisposition = response.headers.get("Content-Disposition"); const isInline = contentDisposition?.split(";")[0] === "inline"; const filename = contentDisposition?.split("filename=")[1]; const url = window.URL.createObjectURL(blob); if (isInline) { window.open(url, "_blank"); } else { const a = document.createElement("a"); a.href = url; a.download = filename || "file.txt"; a.click(); } window.URL.revokeObjectURL(url); };
Uploading as Application/octet-stream is even simpler then using FormData: set the headers with the file details and content type, then add the file to the request body and that's it!
Conclusion
It can be a bit confusing how to view, download and upload files between platforms, in this posts we saw the most common ones.
I hope to have helped you ?
Fallow me on @twitter
The above is the detailed content of React & Expo - How to Upload & Download Files. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


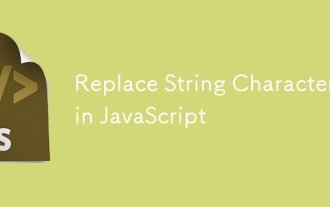
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
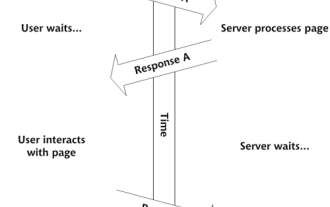
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
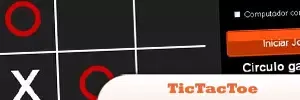
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
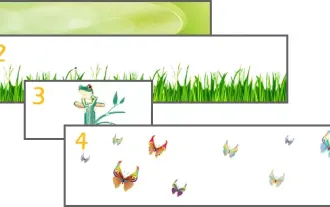
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
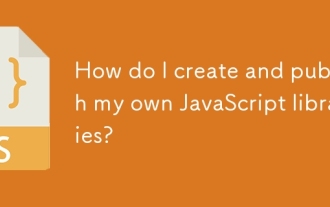
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
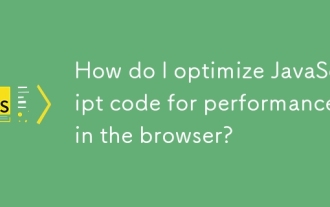
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
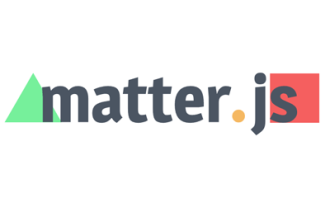
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
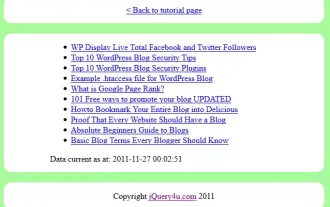
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
