


How Can I Optimize Pygame Event Handling to Prevent Missed or Delayed Events?
Optimizing Event Handling for Pygame Applications
In a quest for a swift-moving Asteroidz clone, a developer encountered bottlenecks in event handling due to delayed and missed events. The code in question consists of two for event in pygame.event.get() loops to monitor exit requests, initiate the game with the spacebar, and restrict rapid-fire bullet shooting.
Crux of the Problem
The problem lies in the utilization of multiple pygame.event.get() loops. By design, this function retrieves all events from the event queue and subsequently removes them. As a result, when multiple loops are employed, only one of them receives the events, leading to potential event loss.
Solution
The key to resolving this issue is to retrieve events only once per frame and then distribute them to various event loops or functions for handling. Here's an optimized implementation:
def handle_events(events): for event in events: # ... Event handling logic ... while run: event_list = pygame.event.get() # ... Code that doesn't require events ... # 1st event loop for event in event_list: # ... Event handling logic ... # ... Code that doesn't require events ... # 2nd event loop for event in event_list: # ... Event handling logic ... # ... Code that doesn't require events ... # Function that handles events handle_events(event_list)
By aggregating all events into a single list and then passing it to the different loops or functions, the events are processed efficiently without any loss. This approach ensures that all event-related code has access to the same up-to-date event information.
The above is the detailed content of How Can I Optimize Pygame Event Handling to Prevent Missed or Delayed Events?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










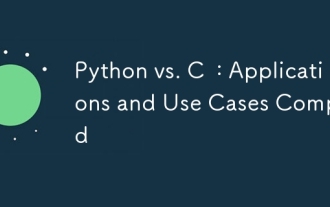
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
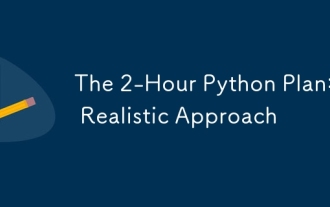
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
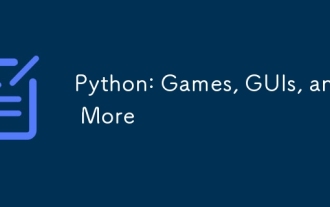
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
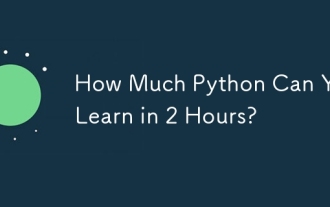
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
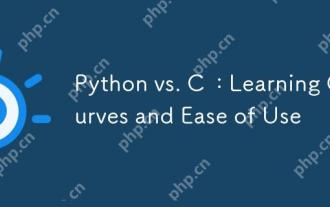
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
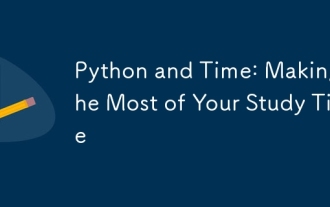
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
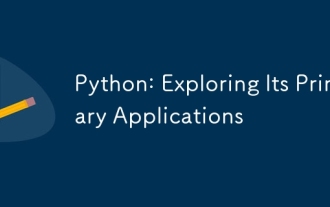
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
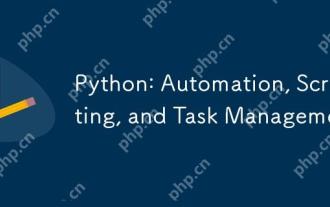
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
