


How Can I Use Tkinter's `after` Method to Create a Timed Animation?
Tkinter: Mastering the 'after' Method for Timed Actions
In the realm of graphical user interfaces, Tkinter stands as a versatile toolkit for Python developers. One particularly useful method within Tkinter is 'after', which enables the execution of code after a specified time interval.
In this post, we'll delve into a common use case for 'after': animating the appearance of random letters at regular intervals. We'll explore the intricacies of the method and guide you through a practical solution.
The Challenge
A budding Python enthusiast encounters trouble using 'frame.after' to generate a random letter every 5 seconds. Their code successfully sets up the application's layout but fails to trigger the animation loop.
The Solution
To address the issue, we introduce a key concept: the 'callback' function. 'after' requires a function as its second parameter, which will be invoked when the time delay expires.
In this scenario, we define a function named 'add_letter'. 'add_letter' randomly selects a letter from a list and renders it as a label within the frame. Crucially, it also re-schedules itself to be called again after the 500 ms delay, thus perpetuating the animation loop.
The Refined Code
tiles_letter = ['a', 'b', 'c', 'd', 'e'] def add_letter(): if not tiles_letter: return rand = random.choice(tiles_letter) tile_frame = Label(frame, text=rand) tile_frame.pack() root.after(500, add_letter) tiles_letter.remove(rand) root.after(0, add_letter) # Initiate animation on start root.mainloop()
Points to Note:
- The 'add_letter' function includes an if statement to check for an empty 'tiles_letter' list. This prevents an exception when all letters have been displayed.
- We initiate the animation by invoking 'root.after(0, add_letter)' immediately after the mainloop starts. This ensures that 'add_letter' is executed as soon as the GUI is ready.
- Recall that the 'after' method only triggers the callback once. By calling 'root.after' inside the 'add_letter' function, we re-register the callback, ensuring continuous execution at the desired time intervals.
Conclusion
Harnessing the power of 'after' opens up a wide range of possibilities for timed actions in your Tkinter applications. With a deep understanding of its syntax and usage, you can create dynamic and engaging interfaces that meet your every need.
The above is the detailed content of How Can I Use Tkinter's `after` Method to Create a Timed Animation?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










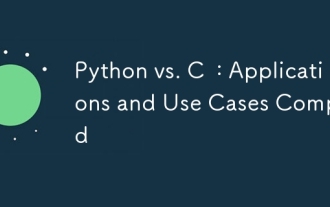
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
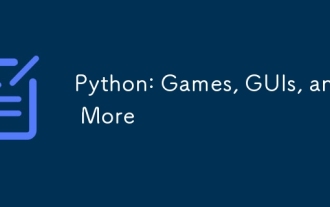
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
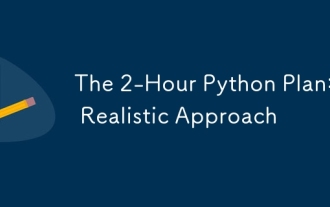
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
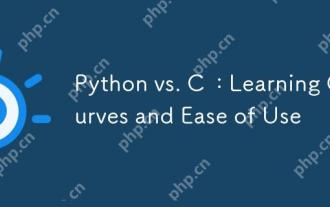
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
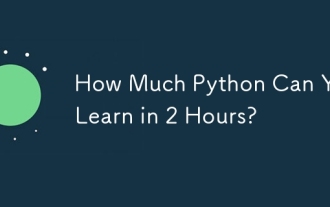
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
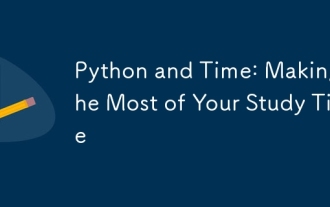
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
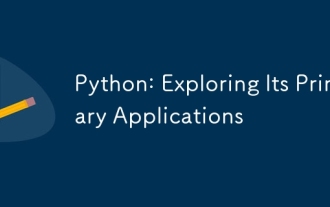
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
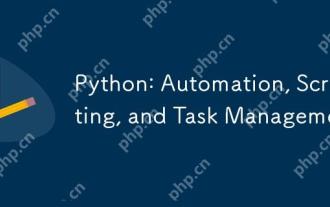
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
