


How to Effectively Test HTTP Calls in Go Using the `httptest` Package?
How to Test HTTP Calls in Go Using httptest
In Go, the httptest package offers a convenient way to test your HTTP calls. It provides both response and server tests for thorough examination of your application's HTTP functionality.
Response Tests
Response tests focus on validating the response itself. For instance, you can verify the response's status code, headers, and content. Here's an example:
func TestHeader3D(t *testing.T) { resp := httptest.NewRecorder() // ... setup the request with headers and parameters ... http.DefaultServeMux.ServeHTTP(resp, req) // ... assert the response body and content type ... }
Server Tests
Server tests, in contrast, enable you to test the entire HTTP server, including its routes and handlers. This approach can be useful for testing the flow of requests through your application. Here's an example using the httptest.NewServer() method:
func TestIt(t *testing.T) { ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // ... your handler setup and response ... })) defer ts.Close() // ... setup your requests and make assertions based on the responses ... }
In your specific case, you can utilize server tests to mock the Twitter search API with a predictable response. This allows you to test your function without making actual HTTP calls.
func TestRetrieveTweets(t *testing.T) { ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Set up your mock response for the Twitter API ... })) defer ts.Close() twitterUrl = ts.URL c := make(chan *twitterResult) go retrieveTweets(c) // ... assert the results you receive in the `c` channel ... }
Remember that the r parameter in your retrieveTweets function is already a pointer, so there's no need to pass it as a pointer within json.Unmarshal.
The above is the detailed content of How to Effectively Test HTTP Calls in Go Using the `httptest` Package?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










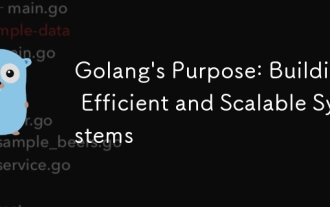
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
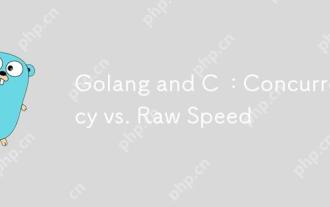
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
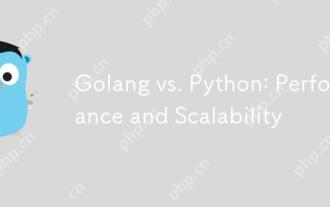
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
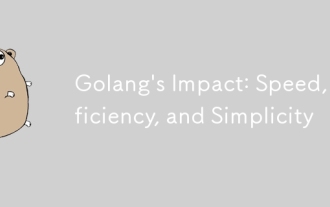
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
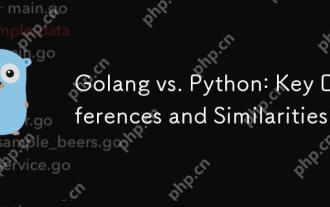
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
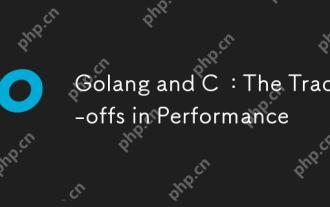
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
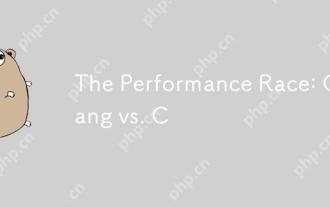
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
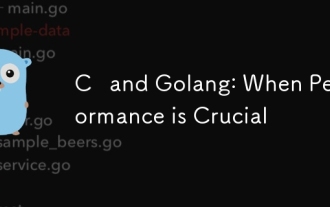
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
