


How Do Go's `Is()` and `As()` Functions Handle Recursive Error Wrapping?
Go Errors: Unraveling the Mysteries of Is() and As()
In Go, error handling is crucial for providing meaningful diagnostics and implementing robust programs. The errors package offers Is() and As() functions for determining error equality and extracting specific details, respectively. One common misconception is that these functions support recursive error wrapping, enabling the unwrapping of multiple nested errors.
However, upon closer inspection, the standard fmt.Errorf function does not provide recursive error wrapping. This means that wrapping errors using %w does not allow for the full traversal of an error chain.
To address this issue, custom error types can be defined that implement the error interface and implement their own Is() and As() methods. This allows for the recursive unwrapping of multiple nested errors.
Example:
type customError struct { err error wrapped *customError } func (c *customError) Error() string { if c.err != nil { return c.err.Error() } return "Custom error without cause" } func (c *customError) Is(err error) bool { if c.err != nil { return errors.Is(c.err, err) } return false } func (c *customError) As(target interface{}) bool { if c.err != nil { return errors.As(c.err, target) } return false } func Wrap(errs ...error) error { var rootError *customError for i := len(errs) - 1; i >= 0; i-- { rootError = &customError{ err: errs[i], wrapped: rootError, } } return rootError }
With this custom error type, you can easily wrap and unwrap multiple errors and perform recursive Is() and As() checks:
err := Wrap(Err1, Err2, Err3) fmt.Println(errors.Is(err, Err1)) // true fmt.Println(errors.Is(err, Err3)) // false var errType ErrType errors.As(err, &errType) fmt.Println(errType) // "my error type"
While this custom implementation provides recursive error unwrapping, there is currently no built-in type in the Go standard library that provides this functionality out of the box. However, libraries like github.com/pkg/errors offer additional error-handling capabilities, including recursive unwrapping, which may be worth considering in your own projects.
The above is the detailed content of How Do Go's `Is()` and `As()` Functions Handle Recursive Error Wrapping?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










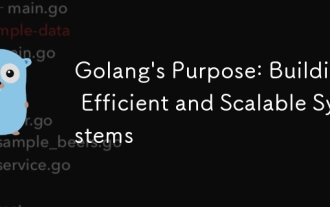
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
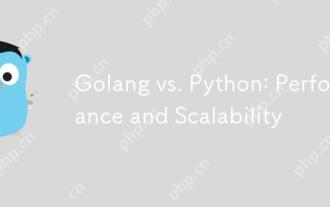
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
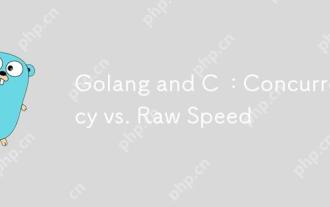
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
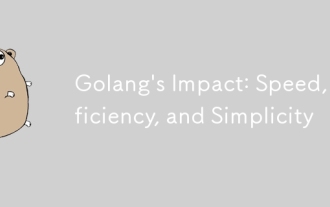
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
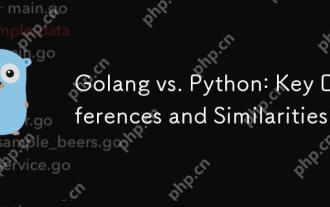
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
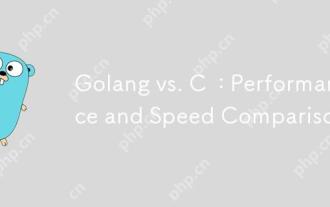
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
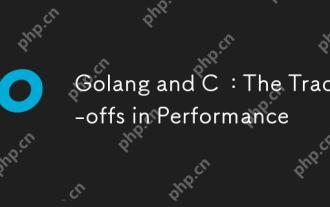
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
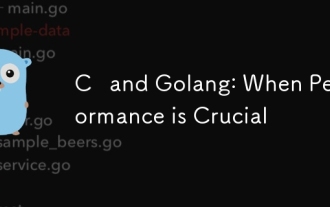
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
