Can I Use Pairs as Keys in C Unordered Maps, and How?
Dec 16, 2024 pm 02:11 PMCan I use Pairs as Keys in Unordered Maps?
In C , you can encounter a compilation error when using an unordered_map with pair as its key. The issue arises from utilizing a custom type as the key, without providing an appropriate hash function for it.
Understanding the Error
The error you encounter is because unordered_map relies on a hashing mechanism to efficiently locate key-value pairs. However, it lacks a default hash function for complex types like pairs. The error message indicates that the compiler cannot implicitly instantiate an undefined hash function for your pair key type.
Solution: Providing a Hash Function
To resolve this issue, you need to define a hash function that calculates a unique hash value for your Vote pair type. This function should accept a pair as an argument and output a hash value.
Here's an example hash function implementation:
struct pair_hash { template<class T1, class T2> std::size_t operator()(const std::pair<T1, T2> &p) const { auto h1 = std::hash<T1>()(p.first); auto h2 = std::hash<T2>()(p.second); return h1 ^ h2; } };
With this hash function, you can modify your code to create an unordered_map that uses pairs as keys:
using Vote = std::pair<std::string, std::string>; using Unordered_map = std::unordered_map<Vote, int, pair_hash>;
Now, you can use this unordered_map as intended, avoiding the compilation error.
The above is the detailed content of Can I Use Pairs as Keys in C Unordered Maps, and How?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
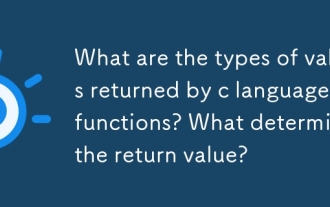
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
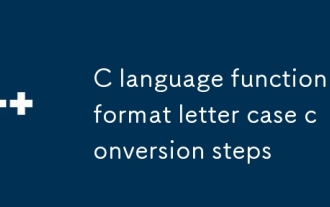
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
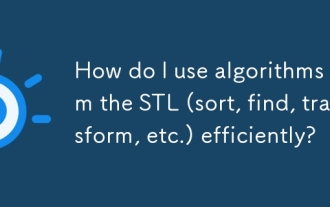
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
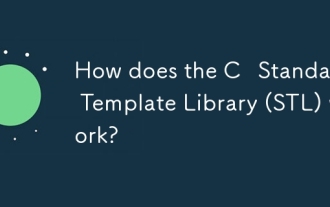
How does the C Standard Template Library (STL) work?
