


How to Split a String Using Spaces While Ignoring Spaces Within Quotes Using Regex?
Regex for Splitting Strings Using Spaces
When working with strings, we often need to split them into individual words for analysis or processing. However, spaces within quoted texts (e.g., "This is a string") should not be considered as separators. Regular expressions (Regex) offer a powerful way to handle such complex splitting tasks.
Question:
Create a Regex expression to split a string using spaces, disregarding spaces surrounded by single or double quotes.
Example:
Input: "This is a string that "will be" highlighted when your 'regular expression' matches something."
Desired Output:
This is a string that will be highlighted when your regular expression matches something.
Answer:
While the provided expression of (?!") does not split correctly, a comprehensive Regex expression can be formulated as follows:
This expression effectively captures two types of elements:
- Unquoted Words: [^s"'] matches sequences of characters without spaces or quotes.
-
Quoted Text:
- /"([^"]*)"/ matches double-quoted text, excluding the quotes.
- /'([^']*)'/ similarly matches single-quoted text, excluding the quotes.
Java Implementation:
The following Java code illustrates how to apply this Regex to split the string:
import java.util.ArrayList; import java.util.List; import java.util.regex.Matcher; import java.util.regex.Pattern; public class RegexSplitter { public static void main(String[] args) { String subjectString = "This is a string that \"will be\" highlighted when your 'regular expression' matches something."; // Pattern that matches unquoted words, quoted texts, and the capturing groups Pattern regex = Pattern.compile("[^\s\"']+|\"([^\"]*)\"|'([^']*)'"); Matcher regexMatcher = regex.matcher(subjectString); // List to store the split words List<String> matchList = new ArrayList<>(); while (regexMatcher.find()) { // Check for capturing groups to exclude quotes if (regexMatcher.group(1) != null) { // Add double-quoted string without the quotes matchList.add(regexMatcher.group(1)); } else if (regexMatcher.group(2) != null) { // Add single-quoted string without the quotes matchList.add(regexMatcher.group(2)); } else { // Add unquoted word matchList.add(regexMatcher.group()); } } // Display the split words for (String word : matchList) { System.out.println(word); } } }
Output:
This is a string that will be highlighted when your regular expression matches something
This enhanced discussion clarifies the problem and provides a more accurate and comprehensive Regex expression, along with a detailed Java implementation to demonstrate its usage.
The above is the detailed content of How to Split a String Using Spaces While Ignoring Spaces Within Quotes Using Regex?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










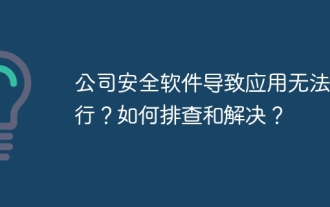
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
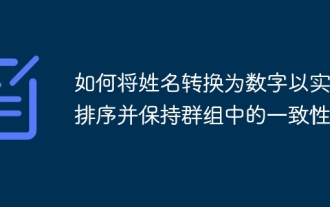
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
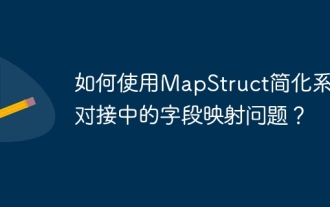
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
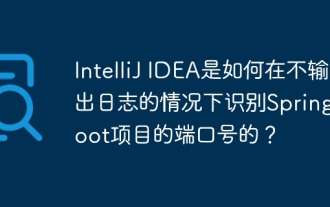
Start Spring using IntelliJIDEAUltimate version...
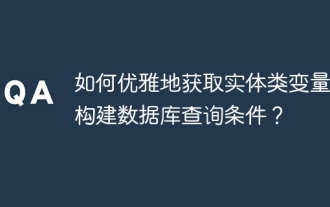
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
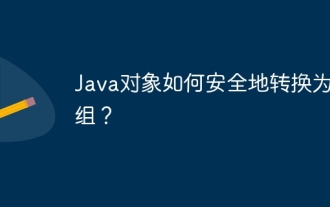
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
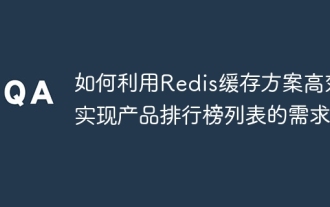
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
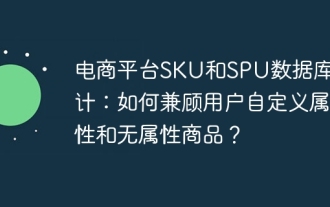
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
