


How Can I Efficiently Retrieve an Element's Initially Defined CSS Property Values?
Extracting Element's CSS Property Values as Set
When needing to obtain the CSS property values of an element as they were initially defined, the function getMatchedStyle offers a solution. This function iterates through the matched CSS rules in descending order of priority, considering both element styles and property importance.
function getMatchedStyle(elem, property){ var val = elem.style.getPropertyValue(property); if(elem.style.getPropertyPriority(property)) return val; var rules = getMatchedCSSRules(elem); for(var i = rules.length; i--;){ var important = r.style.getPropertyPriority(property); if(val == null || important){ val = r.style.getPropertyValue(property); if(important) break; } } return val; }
By considering property importance and element styles, getMatchedStyle accurately returns the CSS property value as set in the stylesheet.
Usage Example
Consider the following HTML and CSS code:
<div class="b">div 1</div> <div>
div { width: 100px; } .d3 { width: auto !important; } div#b { width: 80%; } div#c.c { width: 444px; } x, div.a { width: 50%; } .a { width: 75%; }
Executing the following JavaScript code:
var d = document.querySelectorAll('div'); for(var i = 0; i < d.length; ++i){ console.log("div " + (i+1) + ": " + getMatchedStyle(d[i], 'width')); }
will output:
div 1: 100px div 2: 50% div 3: auto div 4: 44em
This demonstrates the function's ability to accurately extract the set CSS width values of the elements.
The above is the detailed content of How Can I Efficiently Retrieve an Element's Initially Defined CSS Property Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










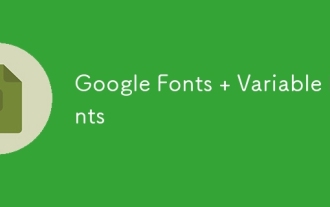
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
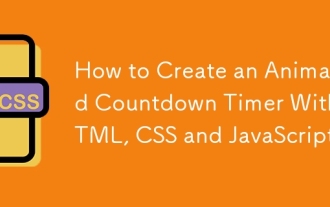
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
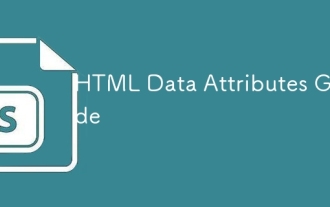
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
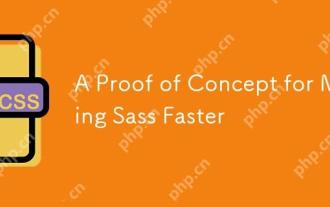
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
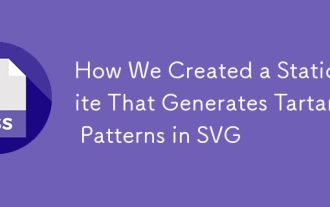
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
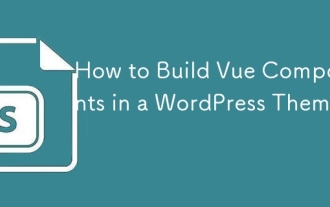
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
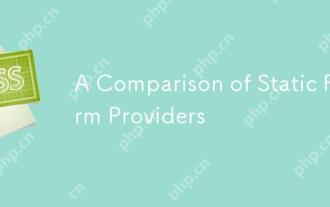
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
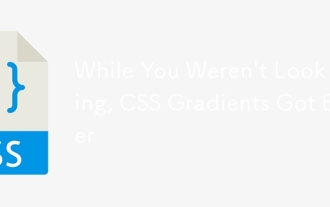
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
