


Does Python Create Copies or References When Assigning Objects?
Does Python Copy Objects on Assignment?
In Python, assignment of variables does not create copies of objects but rather references to them. This behavior can lead to unexpected results.
Example:
Consider the following code:
dict_a = dict_b = dict_c = {} dict_c['hello'] = 'goodbye' print(dict_a) print(dict_b) print(dict_c)
Unexpectedly, this code produces the following output:
{'hello': 'goodbye'} {'hello': 'goodbye'} {'hello': 'goodbye'}
Explanation:
When you assign dict_a = dict_b = dict_c = {}, you are not creating three separate dictionaries. Instead, you are creating one dictionary and assigning three names (references) to it. As a result, any modifications made to one of the references affect all of them.
Solution:
To create independent copies of objects, you can use either the dict.copy() method or copy.deepcopy() function.
Using dict.copy():
dict_a = dict_b.copy() dict_c = dict_b.copy()
Using copy.deepcopy():
import copy dict_a = copy.deepcopy(dict_b) dict_c = copy.deepcopy(dict_b)
The above is the detailed content of Does Python Create Copies or References When Assigning Objects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


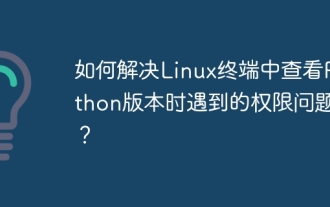
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
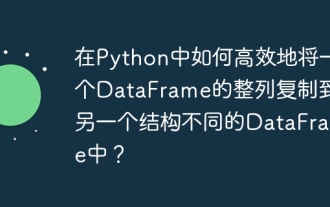
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
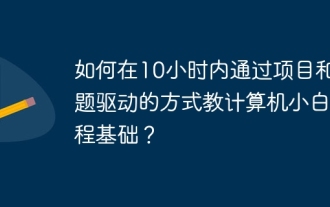
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
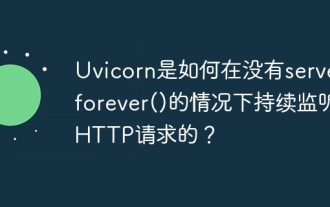
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
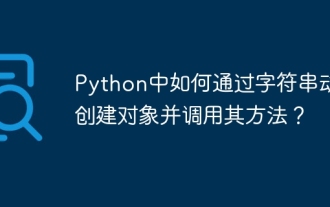
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
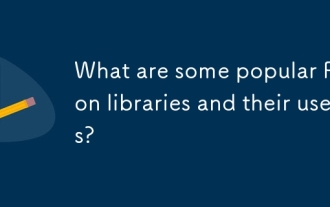
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
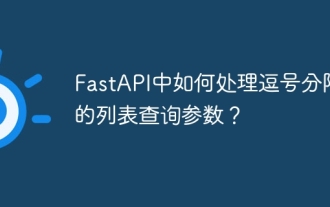
Fastapi ...
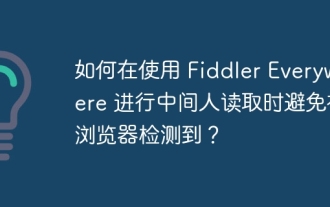
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
