


Why is my Pygame collision detection always True, and why are my sprites positioned at (0, 0)?
Troubleshooting Collision Detection and Sprite Positioning
In your code, you're experiencing two issues: the collide_rect function consistently returns 'True' even when it shouldn't, and the position of the rectangle representing the sprites appears to be (0, 0).
Let's address the collision detection first. The reason why collide_rect always returns 'True' is that you're not setting the position of the rectangles correctly. pygame.Surface.get_rect() returns a rectangle object with the dimensions of the surface, but it always starts at (0, 0). To place the rectangle at the sprite's position, you need to use one of these methods:
self.rect = self.image.get_rect(topleft=(self.x, self.y))
or
self.rect = self.image.get_rect() self.rect.topleft = (self.x, self.y)
By setting the topleft attribute of the rectangle, you're explicitly aligning it with the sprite's position.
As for the incorrect sprite position, you've introduced unnecessary self.x and self.y attributes. It's better practice to rely on the rectangle's position directly. This is an example with the attributes removed and the top-left position being set in the get_rect() call:
class Ball(pygame.sprite.Sprite): def __init__(self): pygame.sprite.Sprite.__init__(self) self.image = pygame.image.load("ball.png") self.rect = self.image.get_rect(topleft=(280, 475)) self.col = False class Obstacle(pygame.sprite.Sprite): def __init__(self): pygame.sprite.Sprite.__init__(self) self.image = pygame.image.load("obstacle.png") self.rect = self.image.get_rect(topleft=(1000, 483))
Additionally, you can opt to utilize a pygame.sprite.Group to handle sprite rendering and updating. This simplifies the drawing process as it draws all the sprites in the group:
all_sprites = pygame.sprite.Group([obstacle, ball]) while not crashed: # [...] gameDisplay.fill((255,255,255)) all_sprites.draw(gameDisplay) pygame.display.flip() clock.tick(1000)
Please note that the Ball.update() and Obstacle.update() methods can be removed if you use a Group as they will handle updating the sprite's position and drawing the image to the display.
The above is the detailed content of Why is my Pygame collision detection always True, and why are my sprites positioned at (0, 0)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
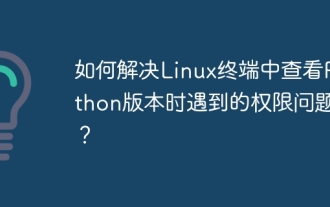
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
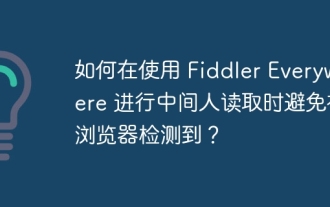
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
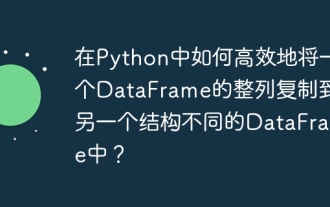
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
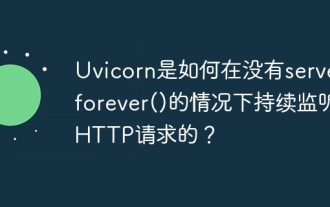
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
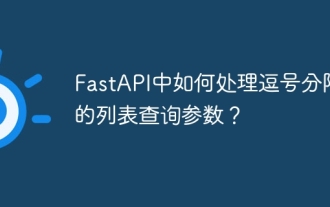
Fastapi ...
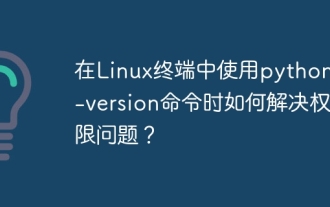
Using python in Linux terminal...
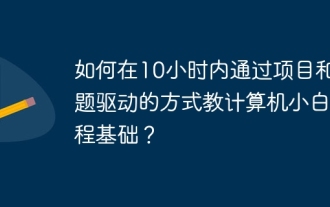
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
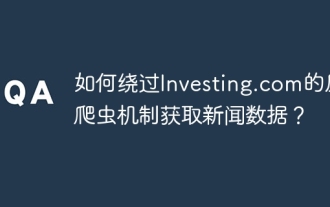
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
