


How to Effectively Mock Filesystem Interactions for Go Unit Testing?
Mocked Filesystem Testing in Go
Introduction
Unit testing functions that interact with the filesystem often requires mocking the filesystem to provide controlled input and verify expected outputs.
Implementation Details
Mocked Interface and File Types
To mock the filesystem, define an interface, fileSystem, that declares methods for file operations like opening and accessing file info, and create a concrete type, osFS, that implements this interface for actual filesystem interactions.
Test Function
As an example, consider getSize(name string), which returns the size of a file given its name using fs.Stat(name) for file information.
Mocked Filesystem Setup
In testing code, replace the global fs variable with a mocked mockedFS that inherits from fileSystem and provides controlled behavior. You can specify whether errors should be reported and the size to be returned by Stat.
Test Code
Use mockedFS to test different scenarios, such as error reporting and size retrieval.
Example Test Code
type mockedFS struct { osFS reportErr bool reportSize int64 } func (m mockedFS) Stat(name string) (os.FileInfo, error) { if m.reportErr { return nil, os.ErrNotExist } return mockedFileInfo{size: m.reportSize}, nil } func TestGetSize(t *testing.T) { oldFs := fs mfs := &mockedFS{} fs = mfs defer func() { fs = oldFs }() mfs.reportErr = true if _, err := getSize("hello.go"); err == nil { t.Error("Expected error, but err is nil!") } mfs.reportErr = false mfs.reportSize = 123 if size, err := getSize("hello.go"); err != nil { t.Errorf("Expected no error, got: %v", err) } else if size != 123 { t.Errorf("Expected size %d, got: %d", 123, size) } }
Conclusion
By creating a mocked filesystem interface, you can control the behavior of filesystem interactions during unit testing, allowing you to thoroughly test code that relies on filesystem operations.
The above is the detailed content of How to Effectively Mock Filesystem Interactions for Go Unit Testing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










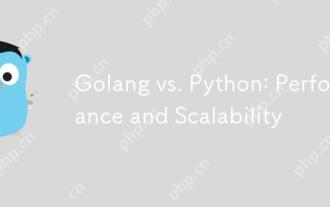
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
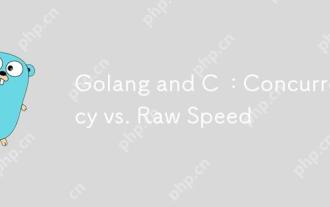
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
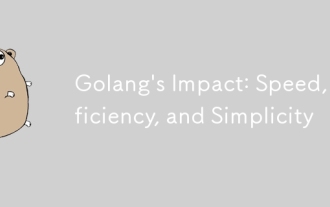
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
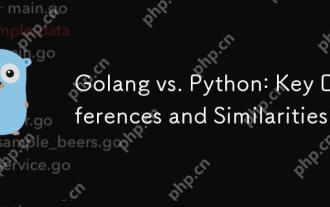
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
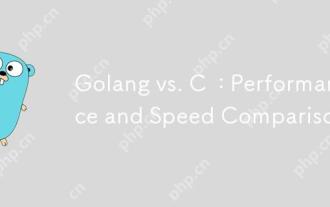
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
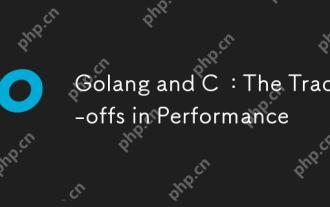
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
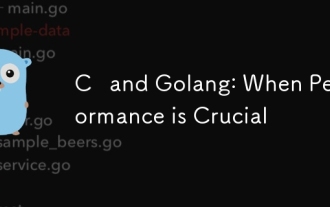
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
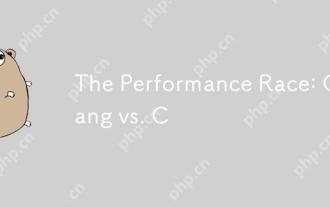
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
