Why Don't Include Guards Prevent Circular #include Issues?
Dec 16, 2024 pm 09:28 PMWhy Don't Include Guards Resolve Circular #include Issues?
Despite using include guards, the issue of circular #includes persists. This is because the preprocessor, which handles #include directives, operates in a manner that exposes the underlying problem.
When the preprocessor encounters an #include statement, it replaces it with the contents of the specified file. For example, if Physics.h includes GameObject.h, the preprocessor would replace the #include "GameObject.h" line in Physics.h with the contents of GameObject.h.
However, if GameObject.h also includes Physics.h, a circular dependency is created. When the preprocessor encounters the #include "Physics.h" line in GameObject.h, it attempts to replace it with the contents of Physics.h, which results in an infinite loop because Physics.h also includes GameObject.h.
To understand this further, consider the following code:
// Physics.h #ifndef PHYSICS_H #define PHYSICS_H #include "GameObject.h" // Guard is present #endif // GameObject.h #ifndef GAMEOBJECT_H #define GAMEOBJECT_H #include "Physics.h" // Guard is also present #endif
When the preprocessor handles this code, it results in the following:
// Physics.h (after preprocessing) #ifndef PHYSICS_H #define PHYSICS_H // (GameObject.h was copy-pasted here) // (GameObject.h was copy-pasted again) #endif // GameObject.h (after preprocessing) #ifndef GAMEOBJECT_H #define GAMEOBJECT_H // (Physics.h was copy-pasted here) // (Physics.h was copy-pasted again) #endif
As you can see, both Physics.h and GameObject.h now contain copies of each other, resulting in a circular dependency.
To resolve this issue, it is crucial to avoid circular #includes and instead use forward declarations. Forward declarations declare the existence of a type without including its definition, allowing the compiler to continue without requiring all the details of the type.
The above is the detailed content of Why Don't Include Guards Prevent Circular #include Issues?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
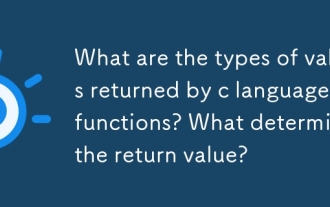
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
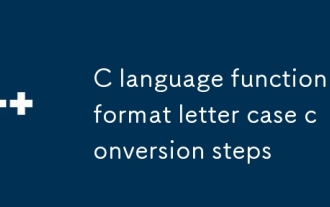
C language function format letter case conversion steps
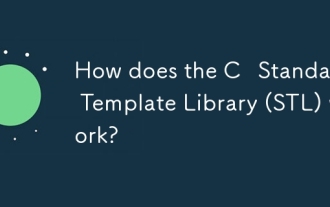
How does the C Standard Template Library (STL) work?
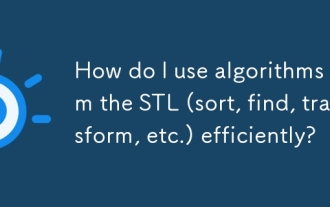
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
