


How to Mock Void Methods in Mockito: A Guide to doThrow, doAnswer, doNothing, and doReturn?
Mocking Void Methods with Mockito
When dealing with observer patterns, mocking methods with void return types can present challenges. One such case is encountering difficulties in mocking a class with a method like addListener, which returns void.
To mock a method with no return value, Mockito provides a set of methods: doThrow(), doAnswer(), doNothing(), and doReturn(). These methods allow you to specify the behavior of the mocked method.
For instance, to specify that the addListener method throws an exception when called, you can use:
Mockito.doThrow(new Exception()).when(instance).addListener(any(Listener.class));
Alternatively, you can chain multiple behaviors together. For example, the following code throws an exception on the first call to addListener and then does nothing on subsequent calls:
Mockito.doThrow(new Exception()).doNothing().when(instance).addListener(any(Listener.class));
In the provided World class, you can mock the setState method using the doAnswer method, as shown below:
World mockWorld = mock(World.class); doAnswer(new Answer<Void>() { public Void answer(InvocationOnMock invocation) { Object[] args = invocation.getArguments(); System.out.println("called with arguments: " + Arrays.toString(args)); return null; } }).when(mockWorld).setState(anyString());
The above is the detailed content of How to Mock Void Methods in Mockito: A Guide to doThrow, doAnswer, doNothing, and doReturn?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










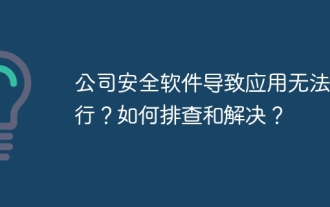
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
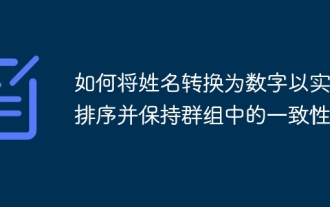
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
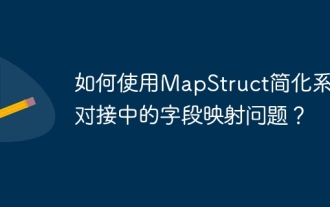
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
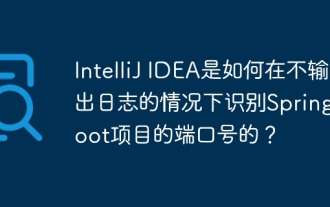
Start Spring using IntelliJIDEAUltimate version...
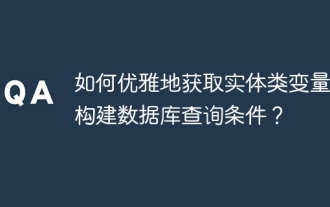
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
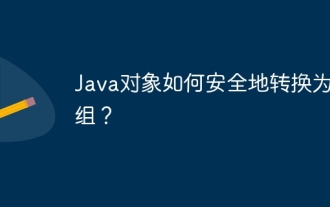
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
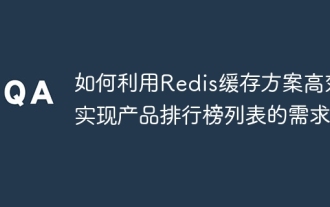
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
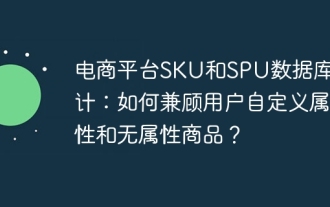
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
