How to Use Multiple JSON Tags for a Single Struct Field in Go?
Multiple JSON Tags for Struct Fields
In a scenario where you receive JSON data from a server and need to unmarshal it into a struct, you might encounter situations where you want to represent the same data with different JSON tags for different purposes.
Customizing JSON Tags
The JSON tag specifies the field name used in the JSON representation. By default, a field's tag is the same as the field name. However, you can customize the tags to change the names used when serializing or deserializing the struct.
Single JSON Tag
Using a single JSON tag is straightforward. Simply specify the desired tag as a string literal:
type Foo struct { Name string `json:"name"` Age int `json:"age"` }
Multiple JSON Tags
As mentioned in the question, it's not possible to attach multiple JSON tags directly to a single field. However, there's a technique that allows you to work around this limitation.
Struct Casting
The given solution suggests using two structs that have the same field layout. For example:
type Foo struct { Name string Age int } type Bar struct { Name string `json:"employee_name"` Age int `json:"-"` }
Then, you can cast the Foo struct to a Bar struct to change the JSON tags. This technique is especially useful when you have a large number of fields:
foo := Foo{Name: "Sam", Age: 20} bar := (*Bar)(unsafe.Pointer(&foo))
Caution
It's important to note that the second struct should be unexported to prevent it from being accessed outside your current package. This ensures that the casting is only performed as intended and not accidentally misused.
Example
The following code demonstrates the casting technique mentioned above:
package main import ( "encoding/json" "fmt" ) type Foo struct { Name string Age int } type Bar struct { Name string `json:"employee_name"` Age int `json:"-"` } func main() { foo := Foo{Name: "Sam", Age: 20} bar := (*Bar)(unsafe.Pointer(&foo)) jsonBytes, err := json.Marshal(bar) if err != nil { fmt.Println(err) return } fmt.Println(string(jsonBytes)) }
This code successfully serializes the Foo struct data using the JSON tags defined in the Bar struct. It produces the following JSON output:
{"employee_name":"Sam"}
The above is the detailed content of How to Use Multiple JSON Tags for a Single Struct Field in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










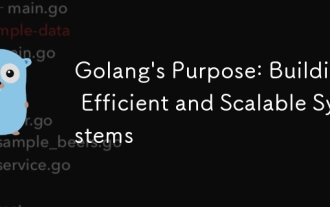
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
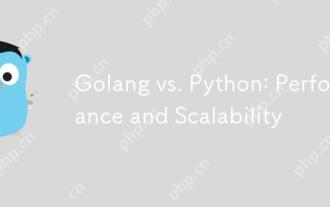
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
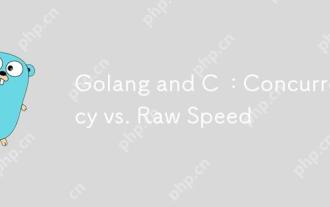
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
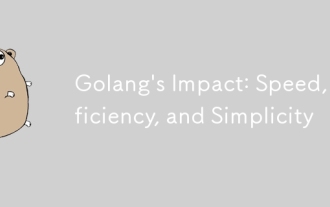
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
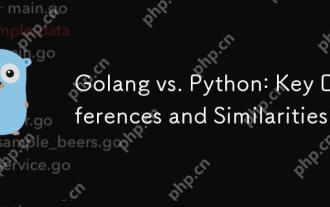
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
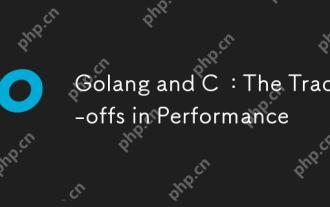
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
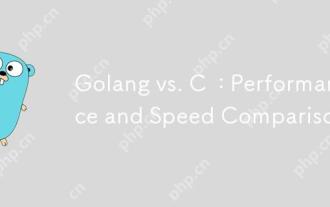
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
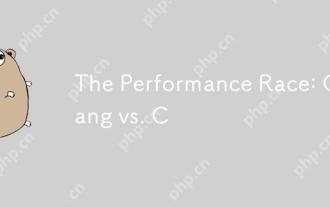
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
