


How Can I Avoid Variable Shadowing Issues When Using Mixed Assignment and Declaration in Go?
Mixed Assignment and Declaration in Go
In Go, when working with variables, it's essential to understand the concept of variable shadowing. This occurs when a variable is declared and initialized in an inner scope with the := operator, creating a new value and type associated with that variable.
Consider the following code snippet:
a := 1 { a, b := 2, 3 }
This code fails to compile because it attempts to redeclare the variable a within the inner scope. However, if we declare both variables in the same scope, it works:
a := 1 a, b := 2, 3
This is a result of variable shadowing. When we use := with a variable within an inner scope, we effectively create a new variable with the same name, which takes precedence over the variable declared in the outer scope.
To resolve this issue, we have several options:
- Declare all variables before using them and assign values with = instead of :=.
- Use different variable names for inner scopes.
- Create a new scope and save the original variable's value before making a new assignment.
Conversely, if we accidentally declare a variable in an inner scope without realizing it (e.g., in a function with multiple return values), we can fix it by:
- Declaring the variables before using them (with =).
- Separating the initial := and statement to allow the variable to be declared as intended.
- Changing all instances of = to :=.
Finally, the code snippet where you combine a new variable declaration (b) with an assignment to an existing variable (a) works because no new scope is created, so the shadowing effect does not occur. You can verify this by printing the address of a before and after the assignment:
a := 1 fmt.Println(&a) a, b := 2, 3 fmt.Println(&a) a = b // Avoids "declared but not used" error for `b`
By understanding variable shadowing and the different ways to both utilize and mitigate it, you can effectively manage variables in Go.
The above is the detailed content of How Can I Avoid Variable Shadowing Issues When Using Mixed Assignment and Declaration in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










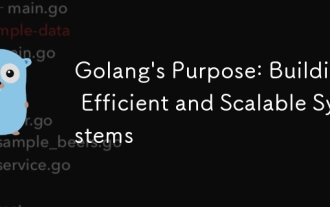
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
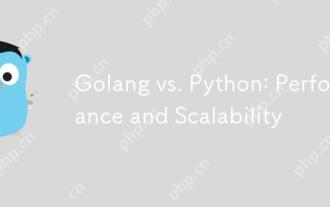
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
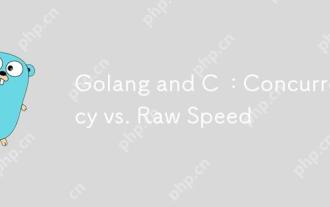
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
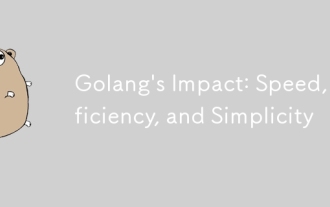
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
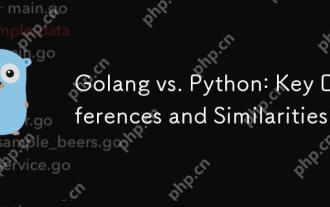
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
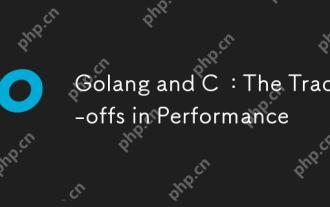
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
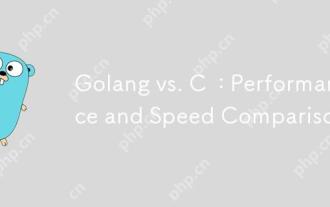
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
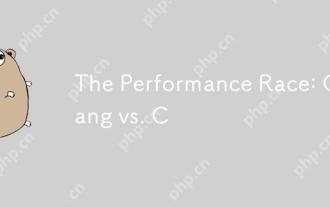
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
