How to Implement Precise Delays in Java Execution?
Pausing Execution in Java for Delay
In Java, executing a loop that requires time-bound execution introduces the need for delays. This guide addresses the method for incorporating delays in Java.
Using TimeUnit for Delay
Leveraging java.util.concurrent.TimeUnit, you can introduce delays as seen below:
TimeUnit.SECONDS.sleep(1); // Pauses for one second TimeUnit.MINUTES.sleep(1); // Pauses for one minute
However, this method may lead to drift over time due to the natural execution variations in a loop.
Alternative with ScheduledExecutorService
For more precise and flexible delays, consider the ScheduledExecutorService. It allows you to schedule tasks with fixed rates or delays:
For Java 8:
final ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor(); executorService.scheduleAtFixedRate(App::myTask, 0, 1, TimeUnit.SECONDS);
For Java 7:
final ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor(); executorService.scheduleAtFixedRate(new Runnable() { @Override public void run() { myTask(); } }, 0, 1, TimeUnit.SECONDS);
The above is the detailed content of How to Implement Precise Delays in Java Execution?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










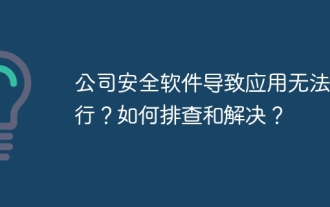
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
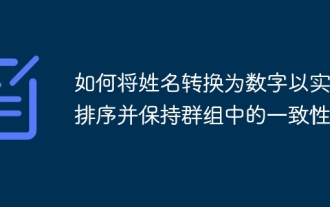
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
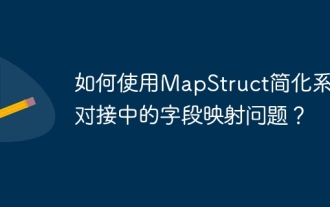
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
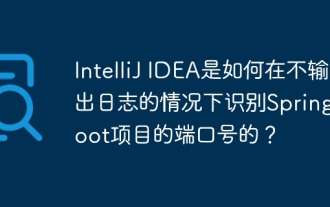
Start Spring using IntelliJIDEAUltimate version...
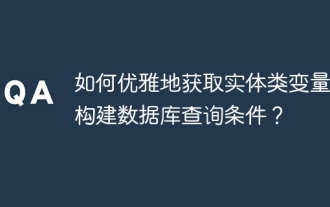
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
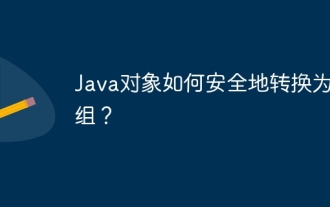
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
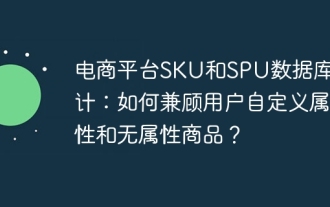
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
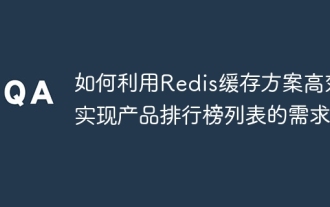
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
