


Why Does My Java Image Resize Result in Low Quality, and How Can I Improve It?
Low Image Quality after Resize in Java
The issue arises when resizing an image from a large size (e.g., 300x300) to a significantly smaller size (e.g., 60x60) in a single step. This inevitably leads to quality degradation.
Divide and Conquer Method for Resizing
To mitigate this problem, it is recommended to use a divide and conquer approach.
- Resize the image to an intermediate size (e.g., 150x150).
- Resize the intermediate image to the final size (e.g., 60x60).
Example Implementation
Here's a Java code snippet that implements the divide and conquer method:
public class ImageResizer { public BufferedImage resize(BufferedImage original, Dimension finalSize) { // Get intermediate size. Dimension intermediateSize = new Dimension(original.getWidth() / 2, original.getHeight() / 2); // Resize to intermediate size. BufferedImage intermediateImage = resize(original, intermediateSize); // Resize to final size. BufferedImage resizedImage = resize(intermediateImage, finalSize); // Return the resized image. return resizedImage; } private BufferedImage resize(BufferedImage image, Dimension size) { BufferedImage scaledImage = new BufferedImage(size.width, size.height, image.getType()); Graphics2D graphics = scaledImage.createGraphics(); // Set rendering hints for better quality. graphics.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR); graphics.setRenderingHint(RenderingHints.KEY_RENDERING, RenderingHints.VALUE_RENDER_QUALITY); graphics.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); // Draw the original image scaled to the new size. graphics.drawImage(image, 0, 0, size.width, size.height, null); // Dispose of the graphics object. graphics.dispose(); // Return the scaled image. return scaledImage; } }
By using this method, you can achieve significantly improved image quality after resizing.
The above is the detailed content of Why Does My Java Image Resize Result in Low Quality, and How Can I Improve It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










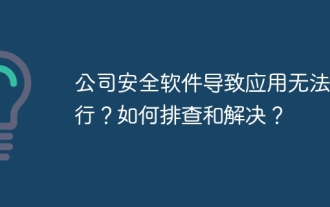
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
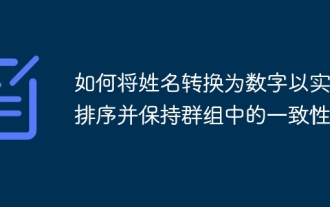
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
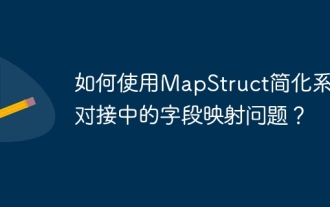
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
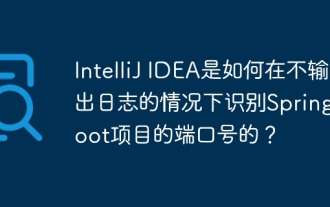
Start Spring using IntelliJIDEAUltimate version...
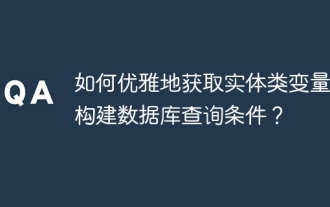
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
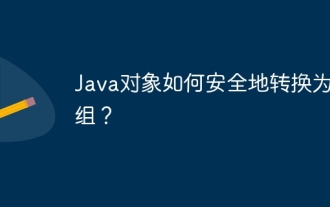
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
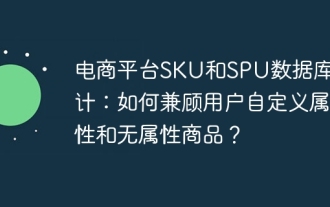
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
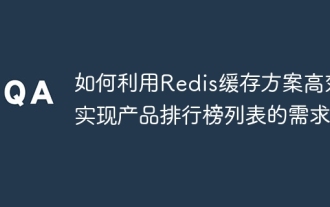
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
