How Can I Efficiently Write Files in Node.js?
File Writing in Node.js: A Comprehensive Guide
Introduction
Node.js empowers developers with robust file system manipulation capabilities. Writing to files is a fundamental task in various application scenarios. This article aims to provide a comprehensive guide to writing to files in Node.js, exploring the File System (fs) API's functionalities and best practices.
Writing to Files
Node.js offers two primary methods for writing to files:
- Asynchronous Writing: Uses the fs.writeFile() function to write data to a file in a non-blocking manner. It accepts the file path, data to write, and a callback function. For example:
const fs = require('fs'); fs.writeFile("/tmp/test", "Hey there!", function(err) { if(err) { return console.log(err); } console.log("The file was saved!"); });
- Synchronous Writing: Uses the fs.writeFileSync() function to write data synchronously. It directly writes data to a file, blocking other operations until writing is complete. For example:
fs.writeFileSync('/tmp/test-sync', 'Hey there!');
Options and Customization
The fs.writeFile() function provides several options to customize file writing:
- Encoding: Specify how the data should be encoded when written to the file, such as 'utf8' or 'base64'.
- Flag: Determine how the file should be opened. The 'w' flag opens the file for writing and truncates any existing content.
- Mode: Set file permissions, such as read-write or read-only, using an octal number.
Best Practices
- Use Streams: For large file writes, consider using the Node.js stream API, which provides a more efficient approach to writing data incrementally.
- Handle Errors: Always handle errors during file writing, as they can occur due to various reasons.
- Close Files: Release file resources by closing open file descriptors after writing is complete.
- Ensure File Permissions: Verify that the Node.js process has the necessary permissions to write to the specified file.
- Test Thoroughly: Perform comprehensive unit testing to ensure file writing functionality across different scenarios and edge cases.
The above is the detailed content of How Can I Efficiently Write Files in Node.js?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










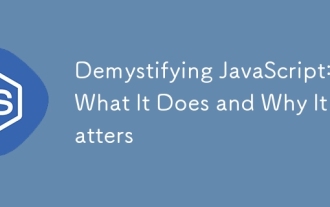
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
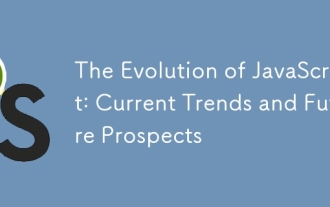
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
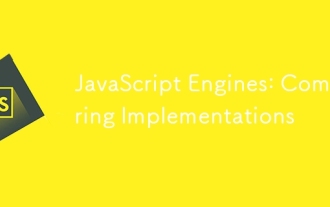
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
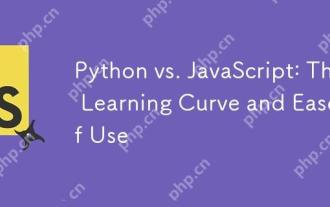
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
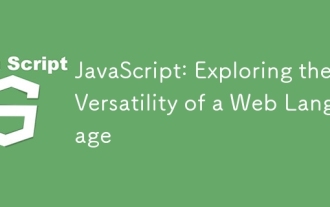
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
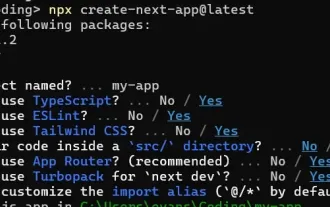
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
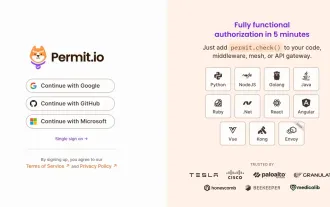
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
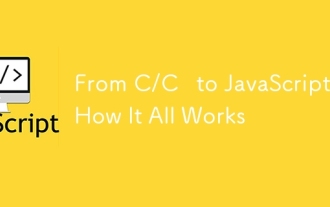
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
