


How to Convert a Pandas DataFrame Column to DateTime Format and Filter by Date?
Transform Pandas DataFrame Column to DateTime Format
Scenario:
Data within a Pandas DataFrame often exists in various formats, including strings. When working with temporal data, timestamps may initially appear as strings but need to be converted to a datetime format for accurate analysis.
Conversion and Filtering Based on Date
To convert a string column to datetime in Pandas, utilize the to_datetime function. This function takes a format argument that specifies the expected format of the string column.
Example:
Consider the following DataFrame with a column (Mycol) containing strings in a custom format:
import pandas as pd raw_data = pd.DataFrame({'Mycol': ['05SEP2014:00:00:00.000']})
To convert this column to datetime, use the following code:
df['Mycol'] = pd.to_datetime(df['Mycol'], format='%d%b%Y:%H:%M:%S.%f')
The format argument specified matches the given string format. After conversion, the Mycol column will now contain datetime objects.
Date-Based Filtering
Once the column is converted to datetime, you can perform date-based filtering operations. For example, to select rows whose date falls within a specific range:
start_date = '01SEP2014' end_date = '30SEP2014' filtered_df = df[(df['Mycol'] >= pd.to_datetime(start_date)) & (df['Mycol'] <= pd.to_datetime(end_date))]
The resulting filtered_df will include only the rows where the Mycol column value is between the specified dates.
The above is the detailed content of How to Convert a Pandas DataFrame Column to DateTime Format and Filter by Date?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




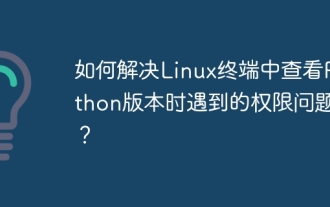
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
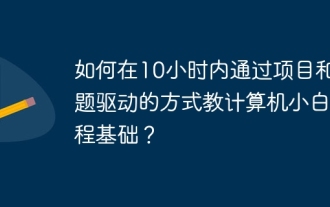
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
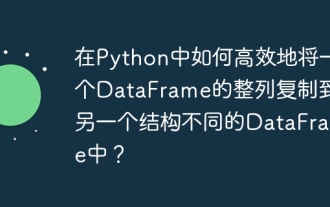
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
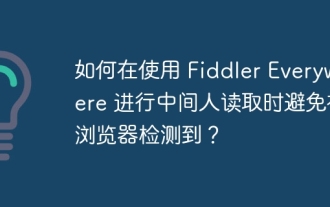
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
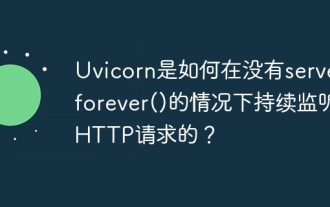
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
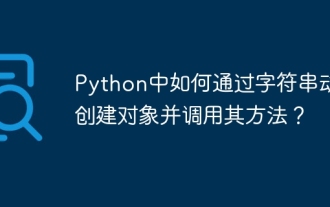
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
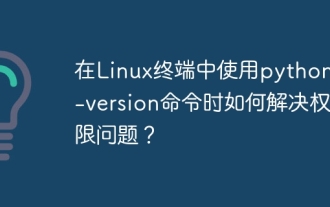
Using python in Linux terminal...
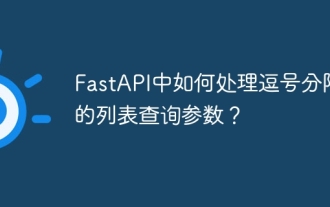
Fastapi ...
