How Can I Iterate Over Unknown Data Structures in Go?
Dec 17, 2024 pm 03:46 PMIterating Over Data Structures in Go Without Knowing Exact Types
Problem:
How can we iterate over a data structure (array or map) in Go without having exact knowledge of its type?
Failed Attempt:
The code below attempts to iterate over an interface representing either a map or an array and execute a function on each item, but it fails due to type checking issues.
func DoTheThingToAllTheThings(data_interface interface{}) int { var numThings int switch data := data_interface.(type) { case map[interface{}]interface{}: numThings = len(data) // ... case []interface{}: numThings = len(data) // ... default: fmt.Println("uh oh!") } return numThings }
Solution:
The fmt.Printf("%vn", data_interface) function provides a way to iterate over the data structure without type-casting.
func PrintData(data_interface interface{}) { fmt.Printf("%v\n", data_interface) }
This works because the %v verb in fmt.Printf uses reflection to determine the type of the argument and print it accordingly.
Reflection in Go:
The fmt.Printf function internally uses the reflect package to inspect the type of the argument and decide how to format it. The reflect.ValueOf(arg) returns a reflect.Value object, which represents the actual value of the argument, and reflect.TypeOf(arg) returns the type of the value.
Example:
The following code reflects a Board struct, then reconstitutes it into a new variable of the same type.
type Board struct { Tboard [9]string Player1 Player Player2 Player } func main() { myBoard := makeBoard() v := reflect.ValueOf(*myBoard) t := v.Type() var b2 Board b2 = v.Interface().(Board) fmt.Printf("v converted back to: %#v\n", b2) }
Note:
In order to use reflection, the data structure's type must beexported, which means it must start with an uppercase letter.
The above is the detailed content of How Can I Iterate Over Unknown Data Structures in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
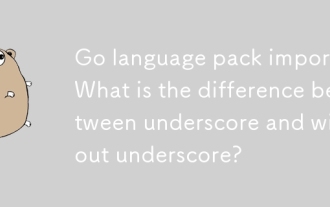
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
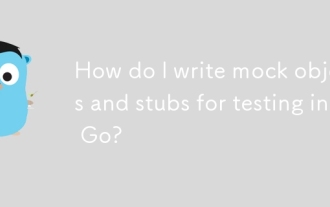
How do I write mock objects and stubs for testing in Go?
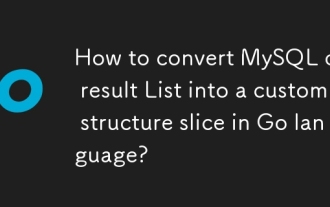
How to convert MySQL query result List into a custom structure slice in Go language?
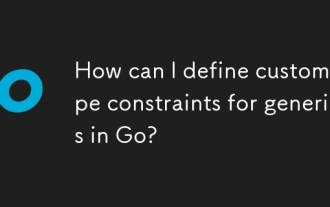
How can I define custom type constraints for generics in Go?
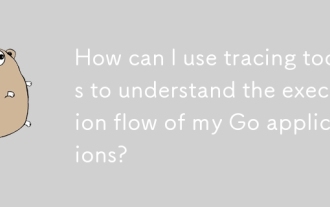
How can I use tracing tools to understand the execution flow of my Go applications?
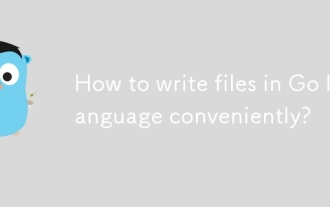
How to write files in Go language conveniently?
