


How to Parse Extremely Large Hexadecimal Strings into Integers in Go with High Precision?
Parsing Long Hexadecimal Strings into uint with High Precision
Converting long hexadecimal strings into decimal notation with high precision is a common task in various programming scenarios. However, the default ParseInt()/ParseUint() functions in Go have limitations when working with numbers that exceed 64-bit precision.
To address this issue, the recommended approach is to utilize the math/big package in Go. This package provides support for working with integers of arbitrary size, enabling you to handle numbers that are far larger than what the standard int64 type can accommodate.
Using math/big for High-Precision Parsing
The math/big package provides a big.Int type that represents integers with arbitrary precision. To convert a hexadecimal string into a big.Int, you can use the following steps:
- Create a new big.Int variable.
- Call the SetString method on the big.Int variable to parse the hexadecimal string. Pass the hexadecimal string as the first argument and the base (16 for hexadecimal) as the second argument.
Example with math/big
Here's an example of how to use math/big to parse a long hexadecimal string into a big.Int:
import ( "fmt" "math/big" ) func main() { s := "0x00000000000000000000000000000000000000000000d3c21bcecceda1000000" i := new(big.Int) i.SetString(s, 16) fmt.Println(i) }
This code converts the provided hexadecimal string into a big.Int and prints the result.
Additional Features of math/big
The math/big package also provides support for the encoding.TextMarshaler and fmt.Scanner interfaces. This allows you to easily read and write big.Int values from and to text-based sources.
For instance, you can use the following syntax to parse a hexadecimal string using fmt.Sscan():
var i big.Int fmt.Sscan("0x000000d3c21bcecceda1000000", &i)
Alternatively, you can use fmt.Sscanf() to parse the hexadecimal string directly into a big.Int:
var i big.Int fmt.Sscanf("0x000000d3c21bcecceda1000000", "0x%x", &i)
The above is the detailed content of How to Parse Extremely Large Hexadecimal Strings into Integers in Go with High Precision?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
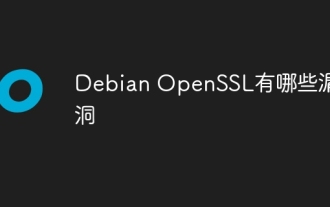
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
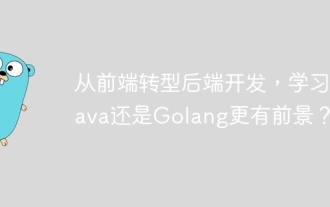
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
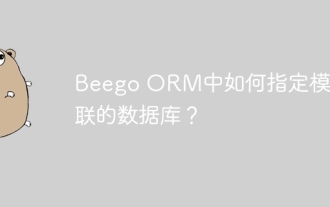
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
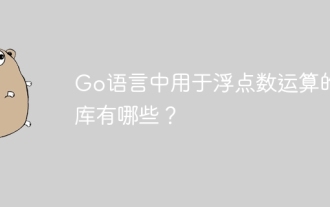
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
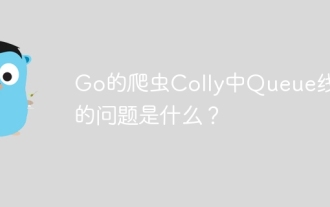
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
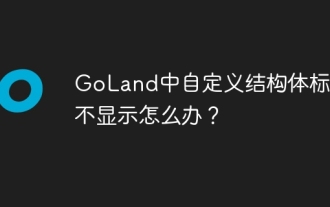
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
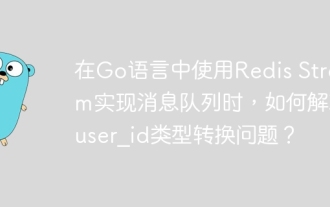
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
