How Can I Optimize Input Scanning in Go to Avoid Timeouts?
Optimizing Input Scanning for Faster Execution
To address the timeout issue experienced with a SPOJ question, specific optimizations are necessary for faster input scanning.
Utilizing bufio.Scanner for Line Reading
Rather than using fmt.Scan alone, leverage bufio.Scanner to efficiently read lines from the input.
Customizing Number Conversion for Speed
Since only numerical input is expected, a custom number converter can be implemented to extract integers directly from raw bytes. This improves performance significantly over using Scanner.Text due to avoiding unnecessary string conversion and overhead.
Custom Number Converter Implementation
The function toInt is designed to convert raw bytes into integers efficiently:
func toInt(buf []byte) (n int) { for _, v := range buf { n = n*10 + int(v-'0') } return }
This function leverages the one-to-one mapping of digits to UTF-8 encoded bytes in the input.
Refined Solution
Combining these optimizations, the improved solution reads as follows:
package main import ( "bufio" "fmt" "os" ) func main() { var n, k, c int scanner := bufio.NewScanner(os.Stdin) scanner.Scan() fmt.Sscanf(scanner.Text(), "%d %d", &n, &k) for ;n > 0; n-- { scanner.Scan() if toInt(scanner.Bytes())%k == 0 { c++ } } fmt.Println(c) }
Benefits of Optimization
This optimized solution significantly improves input scanning speed, ensuring that the program can process the necessary inputs within the allotted time limit. The custom number converter, along with the use of bufio.Scanner, minimizes runtime overhead and enhances program efficiency.
The above is the detailed content of How Can I Optimize Input Scanning in Go to Avoid Timeouts?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


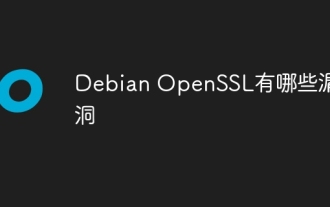
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
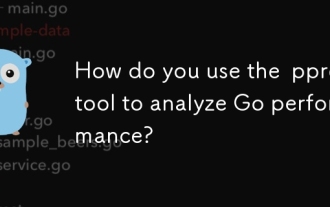
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
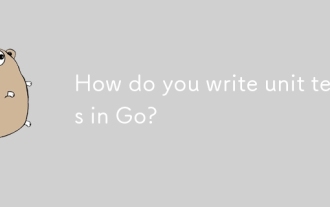
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
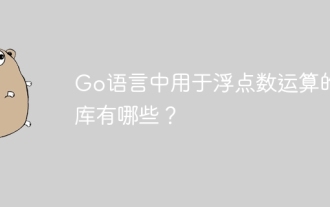
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
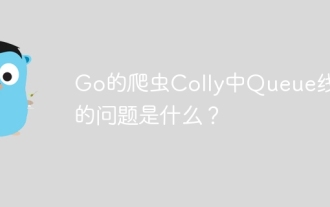
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
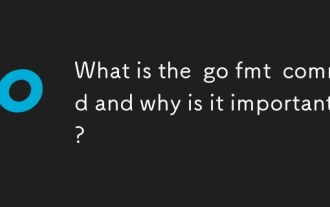
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
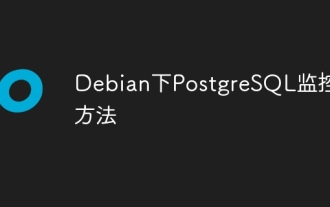
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
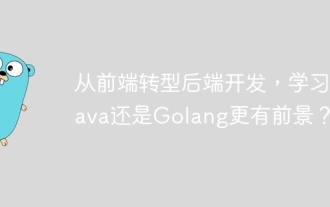
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
