Pandas Boolean Indexing: Why Use `&` Instead of `and`?
Logical Operators for Boolean Indexing in Pandas
When performing Boolean indexing in Pandas, it's crucial to understand the difference between the logical operators & (bitwise AND) and and (logical AND).
Why Use & over and for Boolean Indexing?
Consider the following example:
a = pd.DataFrame({'x': [1, 1], 'y': [10, 20]}) a[(a['x'] == 1) & (a['y'] == 10)]
This code returns the expected result:
x y 0 1 10
However, if you use and instead of &, you'll encounter an error:
a[(a['x'] == 1) and (a['y'] == 10)]
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
Understanding the Error
The error occurs because and tries to evaluate the truthiness of each Series individually (a['x'] and a['y']). However, these Series do not have a clear Boolean value, which leads to the ambiguous truth value error.
In contrast, the bitwise & operator performs element-wise logical operations. It returns a boolean array where each element represents the result of the operation between the corresponding elements in a['x'] and a['y']. This allows you to create a Boolean mask for indexing.
Parentheses: A Mandatory Requirement
Note that it's mandatory to use parentheses when using &. Without them, the operation would be evaluated incorrectly due to the higher operator precedence of & over ==.
a['x'] == 1 & a['y'] == 10 # Incorrect: Triggers the error (a['x'] == 1) & (a['y'] == 10) # Correct: Boolean indexing works as expected
Conclusion
When performing boolean indexing in Pandas, always use the & operator for element-wise logical operations. This ensures proper evaluation and avoids the ambiguous truth value error.
The above is the detailed content of Pandas Boolean Indexing: Why Use `&` Instead of `and`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










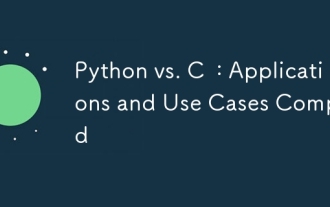
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
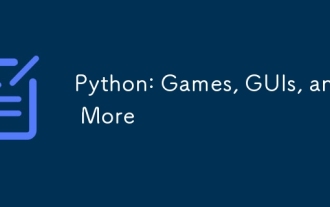
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
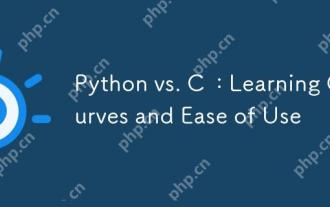
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
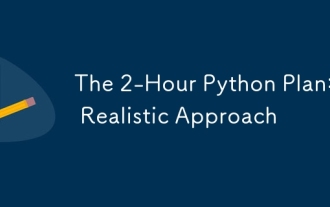
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
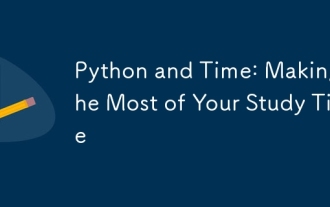
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
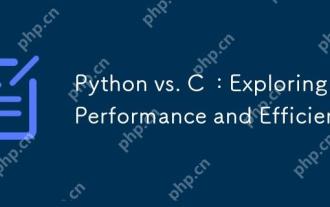
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
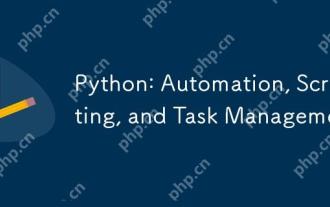
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
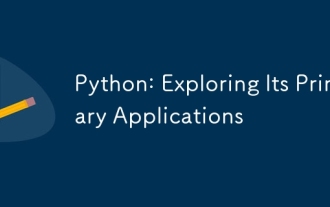
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
