Understanding Local Storage and Session Storage in JavaScript
Local Storage and Session Storage in JavaScript
Web Storage APIs, including Local Storage and Session Storage, provide a simple way to store key-value pairs in a user's browser. These are part of the HTML5 Web Storage specification and are used for persisting data in client-side web applications.
1. Local Storage
- Stores data without expiration.
- Data persists even if the browser is closed and reopened.
Key Features:
- Maximum storage: ~5MB per domain (varies by browser).
- Synchronous API (may block the main thread for large data).
- Accessible only from the same origin.
Common Use Cases:
- Storing user preferences (e.g., theme, language).
- Persisting shopping cart data.
Example:
Storing Data:
localStorage.setItem("username", "JohnDoe");
Retrieving Data:
const username = localStorage.getItem("username"); console.log(username); // Output: JohnDoe
Removing Data:
localStorage.removeItem("username");
Clearing All Data:
localStorage.clear();
2. Session Storage
- Stores data only for the current session.
- Data is cleared when the browser tab or window is closed.
Key Features:
- Maximum storage: ~5MB per domain (varies by browser).
- Synchronous API.
- Accessible only from the same origin and browser session.
Common Use Cases:
- Storing temporary data (e.g., form input during navigation).
- Tracking session-specific preferences.
Example:
Storing Data:
sessionStorage.setItem("isLoggedIn", "true");
Retrieving Data:
const isLoggedIn = sessionStorage.getItem("isLoggedIn"); console.log(isLoggedIn); // Output: true
Removing Data:
sessionStorage.removeItem("isLoggedIn");
Clearing All Data:
sessionStorage.clear();
3. Differences Between Local Storage and Session Storage
Feature | Local Storage | Session Storage | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
Persistent | Cleared after session | |||||||||||||||
Storage Size | ~5MB | ~5MB | |||||||||||||||
Scope | Same-origin policy | Same-origin and session | |||||||||||||||
Use Case | Long-term storage | Temporary/session storage |
4. Storing Complex Data
Both Local Storage and Session Storage store data as strings. To store complex data like objects, you must use JSON.stringify() and JSON.parse().
Example:
localStorage.setItem("username", "JohnDoe");
5. Best Practices
-
Avoid Storing Sensitive Data:
- Data is stored in plain text and can be accessed by JavaScript on the same domain.
- Use secure methods (e.g., HTTP-only cookies) for sensitive data.
-
Check Browser Support:
- Ensure the user's browser supports Local Storage and Session Storage:
const username = localStorage.getItem("username"); console.log(username); // Output: JohnDoe
-
Limit Data Size:
- Store only essential data to avoid performance issues.
-
Use Keys Wisely:
- Use unique keys to prevent conflicts with third-party libraries.
-
Monitor Storage Usage:
- Check available space to avoid exceeding the storage limit.
6. Clearing Storage Programmatically
Example:
localStorage.removeItem("username");
7. Debugging and Managing Storage
Most modern browsers provide developer tools to inspect Local Storage and Session Storage.
Steps:
- Open Developer Tools (F12 or right-click > Inspect).
- Navigate to the "Application" tab.
- Under "Storage," view "Local Storage" and "Session Storage."
8. Summary
Feature | Local Storage | Session Storage | ||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
Yes | No | ||||||||||||
Accessible via JS |
Yes | Yes | ||||||||||||
Data Scope |
Origin | Origin Session |
Local Storage and Session Storage are essential tools for client-side data management. Understanding when to use each and following best practices ensures a secure and efficient implementation in web applications.
The above is the detailed content of Understanding Local Storage and Session Storage in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










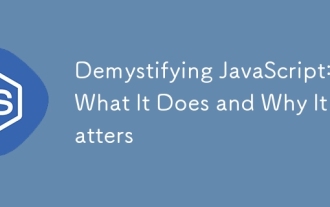
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
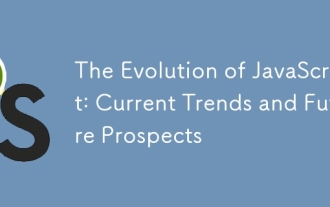
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
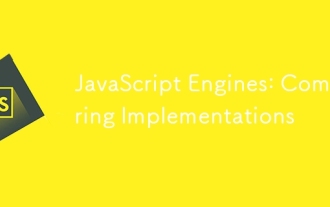
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
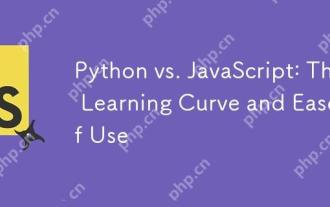
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
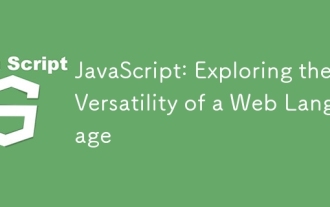
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
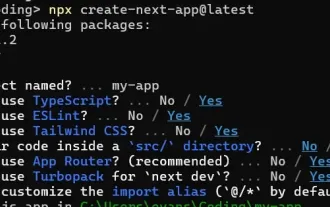
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
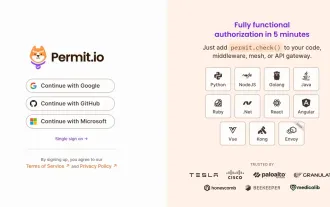
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
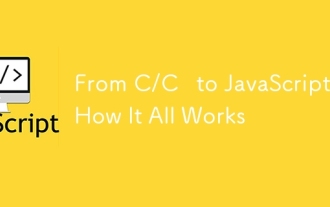
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
